diff --git a/controller/ApiController.php b/controller/ApiController.php
index 4ffb1ab..430d76c 100644
--- a/controller/ApiController.php
+++ b/controller/ApiController.php
@@ -4,6 +4,10 @@
*/
require_once __DIR__ . '/../lib/DirScanner.php';
+//引入验证码类
+require_once __DIR__ . '/../plugins/Captcha/vendor/autoload.php';
+use Gregwar\Captcha\CaptchaBuilder;
+
Class ApiController extends Controller {
protected $version = '1.0';
@@ -76,6 +80,7 @@ Class ApiController extends Controller {
}
//创建目录
+ //创建成功则在data中返回父目录数据结构
public function actionMkdir() {
$code = 0;
$msg = $err = '';
@@ -105,6 +110,12 @@ Class ApiController extends Controller {
try {
$res = mkdir("{$target}/{$newDir}");
if ($res) {
+ //读取父目录数据,并返回包含新建的子目录在内的新数据
+ $scanner = new DirScanner();
+ $scanner->setWebRoot(FSC::$app['config']['content_directory']);
+ $maxLevels = 2;
+ $data = $scanner->scan($target, $maxLevels);
+
$code = 1;
$msg = '目录创建完成';
}else {
@@ -284,4 +295,60 @@ Class ApiController extends Controller {
return $this->renderJson(compact('code', 'msg', 'err', 'data'));
}
+ //验证码图片,data属性里返回图片base64编码格式
+ public function actionCaptcha() {
+ $code = 0;
+ $msg = $err = '';
+ $data = array();
+
+ $refresh = (int)$this->get('refresh', 0);
+
+ try {
+ $builder = new CaptchaBuilder;
+ $builder->build();
+ $captcha_jpg = $builder->get();
+ $captcha_code = $builder->getPhrase();
+ $data = 'data:image/jpeg;base64,' . base64_encode($captcha_jpg);
+ $code = 1;
+ $msg = '验证码图片已生成';
+
+ //save captcha code
+ $userData = $this->getAdmUserData();
+ $userData['captcha_code'] = $captcha_code;
+ $this->saveAdmUserData($userData);
+ }catch(Exception $e) {
+ $err = '验证码图片生成失败:' . $e->getMessage();
+ }
+
+ return $this->renderJson(compact('code', 'msg', 'err', 'data'));
+ }
+
+ //从runtime/admin/目录里获取管理员当前ip相关的缓存数据
+ protected function getAdmUserData() {
+ $data = array();
+
+ $ip = $this->getUserIp();
+ $logDir = __DIR__ . '/../runtime/admin/';
+ $logFile = "{$logDir}{$ip}.cache";
+
+ try {
+ if (file_exists($logFile)) {
+ $data = @json_decode(file_get_contents($logFile), true);
+ }
+ }catch(Exception $e) {}
+
+ return $data;
+ }
+
+ protected function saveAdmUserData($data) {
+ $ip = $this->getUserIp();
+ $logDir = __DIR__ . '/../runtime/admin/';
+ $logFile = "{$logDir}{$ip}.cache";
+ if (!is_dir($logDir)) { //try to mkdir
+ @mkdir($logDir, 0700, true);
+ }
+
+ return @file_put_contents($logFile, json_encode($data));
+ }
+
}
diff --git a/plugins/Captcha/LICENSE b/plugins/Captcha/LICENSE
new file mode 100644
index 0000000..62f991a
--- /dev/null
+++ b/plugins/Captcha/LICENSE
@@ -0,0 +1,19 @@
+Copyright (c) <2012-2017> Grégoire Passault
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is furnished
+to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/plugins/Captcha/README.md b/plugins/Captcha/README.md
new file mode 100644
index 0000000..aaeff98
--- /dev/null
+++ b/plugins/Captcha/README.md
@@ -0,0 +1,146 @@
+Captcha
+=======
+
+
+[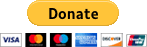](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=YUXRLWHQSWS6L)
+
+Installation
+============
+
+With composer :
+
+``` json
+{
+ ...
+ "require": {
+ "gregwar/captcha": "1.*"
+ }
+}
+```
+
+Usage
+=====
+
+You can create a captcha with the `CaptchaBuilder` :
+
+```php
+build();
+```
+
+You can then save it to a file :
+
+```php
+save('out.jpg');
+```
+
+Or output it directly :
+
+```php
+output();
+```
+
+Or inline it directly in the HTML page:
+
+```php
+
+```
+
+You'll be able to get the code and compare it with a user input :
+
+```php
+getPhrase();
+```
+
+You can compare the phrase with user input:
+```php
+if($builder->testPhrase($userInput)) {
+ // instructions if user phrase is good
+}
+else {
+ // user phrase is wrong
+}
+```
+
+API
+===
+
+You can use theses functions :
+
+* **__construct($phrase = null)**, constructs the builder with the given phrase, if the phrase is null, a random one will be generated
+* **getPhrase()**, allow you to get the phrase contents
+* **setDistortion($distortion)**, enable or disable the distortion, call it before `build()`
+* **isOCRReadable()**, returns `true` if the OCR can be read using the `ocrad` software, you'll need to have shell_exec enabled, imagemagick and ocrad installed
+* **buildAgainstOCR($width = 150, $height = 40, $font = null)**, builds a code until it is not readable by `ocrad`
+* **build($width = 150, $height = 40, $font = null)**, builds a code with the given $width, $height and $font. By default, a random font will be used from the library
+* **save($filename, $quality = 80)**, saves the captcha into a jpeg in the $filename, with the given quality
+* **get($quality = 80)**, returns the jpeg data
+* **output($quality = 80)**, directly outputs the jpeg code to a browser
+* **setBackgroundColor($r, $g, $b)**, sets the background color to force it (this will disable many effects and is not recommended)
+* **setBackgroundImages(array($imagepath1, $imagePath2))**, Sets custom background images to be used as captcha background. It is recommended to disable image effects when passing custom images for background (ignore_all_effects). A random image is selected from the list passed, the full paths to the image files must be passed.
+* **setInterpolation($interpolate)**, enable or disable the interpolation (enabled by default), disabling it will be quicker but the images will look uglier
+* **setIgnoreAllEffects($ignoreAllEffects)**, disable all effects on the captcha image. Recommended to use when passing custom background images for the captcha.
+* **testPhrase($phrase)**, returns true if the given phrase is good
+* **setMaxBehindLines($lines)**, sets the maximum number of lines behind the code
+* **setMaxFrontLines($lines)**, sets the maximum number of lines on the front of the code
+
+If you want to change the number of character, you can call the phrase builder directly using
+extra parameters:
+
+```php
+use Gregwar\Captcha\CaptchaBuilder;
+use Gregwar\Captcha\PhraseBuilder;
+
+// Will build phrases of 3 characters
+$phraseBuilder = new PhraseBuilder(4);
+
+// Will build phrases of 5 characters, only digits
+$phraseBuilder = new PhraseBuilder(5, '0123456789');
+
+// Pass it as first argument of CaptchaBuilder, passing it the phrase
+// builder
+$captcha = new CaptchaBuilder(null, $phraseBuilder);
+```
+
+You can also pass directly the wanted phrase to the builder:
+
+```php
+// Building a Captcha with the "hello" phrase
+$captcha = new CaptchaBuilder('hello');
+```
+
+Complete example
+================
+
+If you want to see an example you can have a look at the ``demo/form.php``, which uses ``demo/session.php`` to
+render a captcha and check it after the submission
+
+Symfony Bundle
+================
+
+You can have a look at the following repository to enjoy the Symfony 2 bundle packaging this captcha generator :
+https://github.com/Gregwar/CaptchaBundle
+
+Yii2 Extension
+===============
+
+You can use the following extension for integrating with Yii2 Framework :
+https://github.com/juliardi/yii2-captcha
+
+License
+=======
+
+This library is under MIT license, have a look to the `LICENSE` file
diff --git a/plugins/Captcha/composer.json b/plugins/Captcha/composer.json
new file mode 100644
index 0000000..4918106
--- /dev/null
+++ b/plugins/Captcha/composer.json
@@ -0,0 +1,19 @@
+{
+ "name": "gregwar/captcha",
+ "type": "library",
+ "description": "Captcha generator",
+ "keywords": ["captcha", "spam", "bot"],
+ "homepage": "https://github.com/Gregwar/Captcha",
+ "license": "MIT",
+ "require": {
+ "php": ">=5.3.0",
+ "ext-gd": "*",
+ "ext-mbstring": "*",
+ "symfony/finder": "*"
+ },
+ "autoload": {
+ "psr-4": {
+ "Gregwar\\": "src/Gregwar"
+ }
+ }
+}
diff --git a/plugins/Captcha/composer.lock b/plugins/Captcha/composer.lock
new file mode 100644
index 0000000..acf57b1
--- /dev/null
+++ b/plugins/Captcha/composer.lock
@@ -0,0 +1,84 @@
+{
+ "_readme": [
+ "This file locks the dependencies of your project to a known state",
+ "Read more about it at https://getcomposer.org/doc/01-basic-usage.md#installing-dependencies",
+ "This file is @generated automatically"
+ ],
+ "content-hash": "86f1eecc42983f38a795e313c72786f6",
+ "packages": [
+ {
+ "name": "symfony/finder",
+ "version": "v6.0.11",
+ "source": {
+ "type": "git",
+ "url": "https://github.com/symfony/finder.git",
+ "reference": "09cb683ba5720385ea6966e5e06be2a34f2568b1"
+ },
+ "dist": {
+ "type": "zip",
+ "url": "https://api.github.com/repos/symfony/finder/zipball/09cb683ba5720385ea6966e5e06be2a34f2568b1",
+ "reference": "09cb683ba5720385ea6966e5e06be2a34f2568b1",
+ "shasum": ""
+ },
+ "require": {
+ "php": ">=8.0.2"
+ },
+ "type": "library",
+ "autoload": {
+ "psr-4": {
+ "Symfony\\Component\\Finder\\": ""
+ },
+ "exclude-from-classmap": [
+ "/Tests/"
+ ]
+ },
+ "notification-url": "https://packagist.org/downloads/",
+ "license": [
+ "MIT"
+ ],
+ "authors": [
+ {
+ "name": "Fabien Potencier",
+ "email": "fabien@symfony.com"
+ },
+ {
+ "name": "Symfony Community",
+ "homepage": "https://symfony.com/contributors"
+ }
+ ],
+ "description": "Finds files and directories via an intuitive fluent interface",
+ "homepage": "https://symfony.com",
+ "support": {
+ "source": "https://github.com/symfony/finder/tree/v6.0.11"
+ },
+ "funding": [
+ {
+ "url": "https://symfony.com/sponsor",
+ "type": "custom"
+ },
+ {
+ "url": "https://github.com/fabpot",
+ "type": "github"
+ },
+ {
+ "url": "https://tidelift.com/funding/github/packagist/symfony/symfony",
+ "type": "tidelift"
+ }
+ ],
+ "time": "2022-07-29T07:39:48+00:00"
+ }
+ ],
+ "packages-dev": [],
+ "aliases": [],
+ "minimum-stability": "stable",
+ "stability-flags": [],
+ "prefer-stable": false,
+ "prefer-lowest": false,
+ "platform": {
+ "php": ">=5.3.0",
+ "ext-gd": "*",
+ "ext-mbstring": "*"
+ },
+ "platform-dev": [],
+ "plugin-api-version": "2.3.0"
+}
diff --git a/plugins/Captcha/src/Gregwar/Captcha/CaptchaBuilder.php b/plugins/Captcha/src/Gregwar/Captcha/CaptchaBuilder.php
new file mode 100644
index 0000000..4d53639
--- /dev/null
+++ b/plugins/Captcha/src/Gregwar/Captcha/CaptchaBuilder.php
@@ -0,0 +1,739 @@
+
+ * @author Jeremy Livingston
+ */
+class CaptchaBuilder implements CaptchaBuilderInterface
+{
+ /**
+ * @var array
+ */
+ protected $fingerprint = array();
+
+ /**
+ * @var bool
+ */
+ protected $useFingerprint = false;
+
+ /**
+ * @var array
+ */
+ protected $textColor = array();
+
+ /**
+ * @var array
+ */
+ protected $lineColor = null;
+
+ /**
+ * @var array
+ */
+ protected $backgroundColor = null;
+
+ /**
+ * @var array
+ */
+ protected $backgroundImages = array();
+
+ /**
+ * @var resource
+ */
+ protected $contents = null;
+
+ /**
+ * @var string
+ */
+ protected $phrase = null;
+
+ /**
+ * @var PhraseBuilderInterface
+ */
+ protected $builder;
+
+ /**
+ * @var bool
+ */
+ protected $distortion = true;
+
+ /**
+ * The maximum number of lines to draw in front of
+ * the image. null - use default algorithm
+ */
+ protected $maxFrontLines = null;
+
+ /**
+ * The maximum number of lines to draw behind
+ * the image. null - use default algorithm
+ */
+ protected $maxBehindLines = null;
+
+ /**
+ * The maximum angle of char
+ */
+ protected $maxAngle = 8;
+
+ /**
+ * The maximum offset of char
+ */
+ protected $maxOffset = 5;
+
+ /**
+ * Is the interpolation enabled ?
+ *
+ * @var bool
+ */
+ protected $interpolation = true;
+
+ /**
+ * Ignore all effects
+ *
+ * @var bool
+ */
+ protected $ignoreAllEffects = false;
+
+ /**
+ * Allowed image types for the background images
+ *
+ * @var array
+ */
+ protected $allowedBackgroundImageTypes = array('image/png', 'image/jpeg', 'image/gif');
+
+ /**
+ * The image contents
+ */
+ public function getContents()
+ {
+ return $this->contents;
+ }
+
+ /**
+ * Enable/Disables the interpolation
+ *
+ * @param $interpolate bool True to enable, false to disable
+ *
+ * @return CaptchaBuilder
+ */
+ public function setInterpolation($interpolate = true)
+ {
+ $this->interpolation = $interpolate;
+
+ return $this;
+ }
+
+ /**
+ * Temporary dir, for OCR check
+ */
+ public $tempDir = 'temp/';
+
+ public function __construct($phrase = null, PhraseBuilderInterface $builder = null)
+ {
+ if ($builder === null) {
+ $this->builder = new PhraseBuilder;
+ } else {
+ $this->builder = $builder;
+ }
+
+ $this->phrase = is_string($phrase) ? $phrase : $this->builder->build($phrase);
+ }
+
+ /**
+ * Setting the phrase
+ */
+ public function setPhrase($phrase)
+ {
+ $this->phrase = (string) $phrase;
+ }
+
+ /**
+ * Enables/disable distortion
+ */
+ public function setDistortion($distortion)
+ {
+ $this->distortion = (bool) $distortion;
+
+ return $this;
+ }
+
+ public function setMaxBehindLines($maxBehindLines)
+ {
+ $this->maxBehindLines = $maxBehindLines;
+
+ return $this;
+ }
+
+ public function setMaxFrontLines($maxFrontLines)
+ {
+ $this->maxFrontLines = $maxFrontLines;
+
+ return $this;
+ }
+
+ public function setMaxAngle($maxAngle)
+ {
+ $this->maxAngle = $maxAngle;
+
+ return $this;
+ }
+
+ public function setMaxOffset($maxOffset)
+ {
+ $this->maxOffset = $maxOffset;
+
+ return $this;
+ }
+
+ /**
+ * Gets the captcha phrase
+ */
+ public function getPhrase()
+ {
+ return $this->phrase;
+ }
+
+ /**
+ * Returns true if the given phrase is good
+ */
+ public function testPhrase($phrase)
+ {
+ return ($this->builder->niceize($phrase) == $this->builder->niceize($this->getPhrase()));
+ }
+
+ /**
+ * Instantiation
+ */
+ public static function create($phrase = null)
+ {
+ return new self($phrase);
+ }
+
+ /**
+ * Sets the text color to use
+ */
+ public function setTextColor($r, $g, $b)
+ {
+ $this->textColor = array($r, $g, $b);
+
+ return $this;
+ }
+
+ /**
+ * Sets the background color to use
+ */
+ public function setBackgroundColor($r, $g, $b)
+ {
+ $this->backgroundColor = array($r, $g, $b);
+
+ return $this;
+ }
+
+ public function setLineColor($r, $g, $b)
+ {
+ $this->lineColor = array($r, $g, $b);
+
+ return $this;
+ }
+
+ /**
+ * Sets the ignoreAllEffects value
+ *
+ * @param bool $ignoreAllEffects
+ * @return CaptchaBuilder
+ */
+ public function setIgnoreAllEffects($ignoreAllEffects)
+ {
+ $this->ignoreAllEffects = $ignoreAllEffects;
+
+ return $this;
+ }
+
+ /**
+ * Sets the list of background images to use (one image is randomly selected)
+ */
+ public function setBackgroundImages(array $backgroundImages)
+ {
+ $this->backgroundImages = $backgroundImages;
+
+ return $this;
+ }
+
+ /**
+ * Draw lines over the image
+ */
+ protected function drawLine($image, $width, $height, $tcol = null)
+ {
+ if ($this->lineColor === null) {
+ $red = $this->rand(100, 255);
+ $green = $this->rand(100, 255);
+ $blue = $this->rand(100, 255);
+ } else {
+ $red = $this->lineColor[0];
+ $green = $this->lineColor[1];
+ $blue = $this->lineColor[2];
+ }
+
+ if ($tcol === null) {
+ $tcol = imagecolorallocate($image, $red, $green, $blue);
+ }
+
+ if ($this->rand(0, 1)) { // Horizontal
+ $Xa = $this->rand(0, $width/2);
+ $Ya = $this->rand(0, $height);
+ $Xb = $this->rand($width/2, $width);
+ $Yb = $this->rand(0, $height);
+ } else { // Vertical
+ $Xa = $this->rand(0, $width);
+ $Ya = $this->rand(0, $height/2);
+ $Xb = $this->rand(0, $width);
+ $Yb = $this->rand($height/2, $height);
+ }
+ imagesetthickness($image, $this->rand(1, 3));
+ imageline($image, $Xa, $Ya, $Xb, $Yb, $tcol);
+ }
+
+ /**
+ * Apply some post effects
+ */
+ protected function postEffect($image)
+ {
+ if (!function_exists('imagefilter')) {
+ return;
+ }
+
+ if ($this->backgroundColor != null || $this->textColor != null) {
+ return;
+ }
+
+ // Negate ?
+ if ($this->rand(0, 1) == 0) {
+ imagefilter($image, IMG_FILTER_NEGATE);
+ }
+
+ // Edge ?
+ if ($this->rand(0, 10) == 0) {
+ imagefilter($image, IMG_FILTER_EDGEDETECT);
+ }
+
+ // Contrast
+ imagefilter($image, IMG_FILTER_CONTRAST, $this->rand(-50, 10));
+
+ // Colorize
+ if ($this->rand(0, 5) == 0) {
+ imagefilter($image, IMG_FILTER_COLORIZE, $this->rand(-80, 50), $this->rand(-80, 50), $this->rand(-80, 50));
+ }
+ }
+
+ /**
+ * Writes the phrase on the image
+ */
+ protected function writePhrase($image, $phrase, $font, $width, $height)
+ {
+ $length = mb_strlen($phrase);
+ if ($length === 0) {
+ return \imagecolorallocate($image, 0, 0, 0);
+ }
+
+ // Gets the text size and start position
+ $size = $width / $length - $this->rand(0, 3) - 1;
+ $box = \imagettfbbox($size, 0, $font, $phrase);
+ $textWidth = $box[2] - $box[0];
+ $textHeight = $box[1] - $box[7];
+ $x = ($width - $textWidth) / 2;
+ $y = ($height - $textHeight) / 2 + $size;
+
+ if (!$this->textColor) {
+ $textColor = array($this->rand(0, 150), $this->rand(0, 150), $this->rand(0, 150));
+ } else {
+ $textColor = $this->textColor;
+ }
+ $col = \imagecolorallocate($image, $textColor[0], $textColor[1], $textColor[2]);
+
+ // Write the letters one by one, with random angle
+ for ($i=0; $i<$length; $i++) {
+ $symbol = mb_substr($phrase, $i, 1);
+ $box = \imagettfbbox($size, 0, $font, $symbol);
+ $w = $box[2] - $box[0];
+ $angle = $this->rand(-$this->maxAngle, $this->maxAngle);
+ $offset = $this->rand(-$this->maxOffset, $this->maxOffset);
+ \imagettftext($image, $size, $angle, $x, $y + $offset, $col, $font, $symbol);
+ $x += $w;
+ }
+
+ return $col;
+ }
+
+ /**
+ * Try to read the code against an OCR
+ */
+ public function isOCRReadable()
+ {
+ if (!is_dir($this->tempDir)) {
+ @mkdir($this->tempDir, 0755, true);
+ }
+
+ $tempj = $this->tempDir . uniqid('captcha', true) . '.jpg';
+ $tempp = $this->tempDir . uniqid('captcha', true) . '.pgm';
+
+ $this->save($tempj);
+ shell_exec("convert $tempj $tempp");
+ $value = trim(strtolower(shell_exec("ocrad $tempp")));
+
+ @unlink($tempj);
+ @unlink($tempp);
+
+ return $this->testPhrase($value);
+ }
+
+ /**
+ * Builds while the code is readable against an OCR
+ */
+ public function buildAgainstOCR($width = 150, $height = 40, $font = null, $fingerprint = null)
+ {
+ do {
+ $this->build($width, $height, $font, $fingerprint);
+ } while ($this->isOCRReadable());
+ }
+
+ /**
+ * Generate the image
+ */
+ public function build($width = 150, $height = 40, $font = null, $fingerprint = null)
+ {
+ if (null !== $fingerprint) {
+ $this->fingerprint = $fingerprint;
+ $this->useFingerprint = true;
+ } else {
+ $this->fingerprint = array();
+ $this->useFingerprint = false;
+ }
+
+ if ($font === null) {
+ $font = __DIR__ . '/Font/captcha'.$this->rand(0, 5).'.ttf';
+ }
+
+ if (empty($this->backgroundImages)) {
+ // if background images list is not set, use a color fill as a background
+ $image = imagecreatetruecolor($width, $height);
+ if ($this->backgroundColor == null) {
+ $bg = imagecolorallocate($image, $this->rand(200, 255), $this->rand(200, 255), $this->rand(200, 255));
+ } else {
+ $color = $this->backgroundColor;
+ $bg = imagecolorallocate($image, $color[0], $color[1], $color[2]);
+ }
+ $this->background = $bg;
+ imagefill($image, 0, 0, $bg);
+ } else {
+ // use a random background image
+ $randomBackgroundImage = $this->backgroundImages[rand(0, count($this->backgroundImages)-1)];
+
+ $imageType = $this->validateBackgroundImage($randomBackgroundImage);
+
+ $image = $this->createBackgroundImageFromType($randomBackgroundImage, $imageType);
+ }
+
+ // Apply effects
+ if (!$this->ignoreAllEffects) {
+ $square = $width * $height;
+ $effects = $this->rand($square/3000, $square/2000);
+
+ // set the maximum number of lines to draw in front of the text
+ if ($this->maxBehindLines != null && $this->maxBehindLines > 0) {
+ $effects = min($this->maxBehindLines, $effects);
+ }
+
+ if ($this->maxBehindLines !== 0) {
+ for ($e = 0; $e < $effects; $e++) {
+ $this->drawLine($image, $width, $height);
+ }
+ }
+ }
+
+ // Write CAPTCHA text
+ $color = $this->writePhrase($image, $this->phrase, $font, $width, $height);
+
+ // Apply effects
+ if (!$this->ignoreAllEffects) {
+ $square = $width * $height;
+ $effects = $this->rand($square/3000, $square/2000);
+
+ // set the maximum number of lines to draw in front of the text
+ if ($this->maxFrontLines != null && $this->maxFrontLines > 0) {
+ $effects = min($this->maxFrontLines, $effects);
+ }
+
+ if ($this->maxFrontLines !== 0) {
+ for ($e = 0; $e < $effects; $e++) {
+ $this->drawLine($image, $width, $height, $color);
+ }
+ }
+ }
+
+ // Distort the image
+ if ($this->distortion && !$this->ignoreAllEffects) {
+ $image = $this->distort($image, $width, $height, $bg);
+ }
+
+ // Post effects
+ if (!$this->ignoreAllEffects) {
+ $this->postEffect($image);
+ }
+
+ $this->contents = $image;
+
+ return $this;
+ }
+
+ /**
+ * Distorts the image
+ */
+ public function distort($image, $width, $height, $bg)
+ {
+ $contents = imagecreatetruecolor($width, $height);
+ $X = $this->rand(0, $width);
+ $Y = $this->rand(0, $height);
+ $phase = $this->rand(0, 10);
+ $scale = 1.1 + $this->rand(0, 10000) / 30000;
+ for ($x = 0; $x < $width; $x++) {
+ for ($y = 0; $y < $height; $y++) {
+ $Vx = $x - $X;
+ $Vy = $y - $Y;
+ $Vn = sqrt($Vx * $Vx + $Vy * $Vy);
+
+ if ($Vn != 0) {
+ $Vn2 = $Vn + 4 * sin($Vn / 30);
+ $nX = $X + ($Vx * $Vn2 / $Vn);
+ $nY = $Y + ($Vy * $Vn2 / $Vn);
+ } else {
+ $nX = $X;
+ $nY = $Y;
+ }
+ $nY = $nY + $scale * sin($phase + $nX * 0.2);
+
+ if ($this->interpolation) {
+ $p = $this->interpolate(
+ $nX - floor($nX),
+ $nY - floor($nY),
+ $this->getCol($image, floor($nX), floor($nY), $bg),
+ $this->getCol($image, ceil($nX), floor($nY), $bg),
+ $this->getCol($image, floor($nX), ceil($nY), $bg),
+ $this->getCol($image, ceil($nX), ceil($nY), $bg)
+ );
+ } else {
+ $p = $this->getCol($image, round($nX), round($nY), $bg);
+ }
+
+ if ($p == 0) {
+ $p = $bg;
+ }
+
+ imagesetpixel($contents, $x, $y, $p);
+ }
+ }
+
+ return $contents;
+ }
+
+ /**
+ * Saves the Captcha to a jpeg file
+ */
+ public function save($filename, $quality = 90)
+ {
+ imagejpeg($this->contents, $filename, $quality);
+ }
+
+ /**
+ * Gets the image GD
+ */
+ public function getGd()
+ {
+ return $this->contents;
+ }
+
+ /**
+ * Gets the image contents
+ */
+ public function get($quality = 90)
+ {
+ ob_start();
+ $this->output($quality);
+
+ return ob_get_clean();
+ }
+
+ /**
+ * Gets the HTML inline base64
+ */
+ public function inline($quality = 90)
+ {
+ return 'data:image/jpeg;base64,' . base64_encode($this->get($quality));
+ }
+
+ /**
+ * Outputs the image
+ */
+ public function output($quality = 90)
+ {
+ imagejpeg($this->contents, null, $quality);
+ }
+
+ /**
+ * @return array
+ */
+ public function getFingerprint()
+ {
+ return $this->fingerprint;
+ }
+
+ /**
+ * Returns a random number or the next number in the
+ * fingerprint
+ */
+ protected function rand($min, $max)
+ {
+ if (!is_array($this->fingerprint)) {
+ $this->fingerprint = array();
+ }
+
+ if ($this->useFingerprint) {
+ $value = current($this->fingerprint);
+ next($this->fingerprint);
+ } else {
+ $value = mt_rand($min, $max);
+ $this->fingerprint[] = $value;
+ }
+
+ return $value;
+ }
+
+ /**
+ * @param $x
+ * @param $y
+ * @param $nw
+ * @param $ne
+ * @param $sw
+ * @param $se
+ *
+ * @return int
+ */
+ protected function interpolate($x, $y, $nw, $ne, $sw, $se)
+ {
+ list($r0, $g0, $b0) = $this->getRGB($nw);
+ list($r1, $g1, $b1) = $this->getRGB($ne);
+ list($r2, $g2, $b2) = $this->getRGB($sw);
+ list($r3, $g3, $b3) = $this->getRGB($se);
+
+ $cx = 1.0 - $x;
+ $cy = 1.0 - $y;
+
+ $m0 = $cx * $r0 + $x * $r1;
+ $m1 = $cx * $r2 + $x * $r3;
+ $r = (int) ($cy * $m0 + $y * $m1);
+
+ $m0 = $cx * $g0 + $x * $g1;
+ $m1 = $cx * $g2 + $x * $g3;
+ $g = (int) ($cy * $m0 + $y * $m1);
+
+ $m0 = $cx * $b0 + $x * $b1;
+ $m1 = $cx * $b2 + $x * $b3;
+ $b = (int) ($cy * $m0 + $y * $m1);
+
+ return ($r << 16) | ($g << 8) | $b;
+ }
+
+ /**
+ * @param $image
+ * @param $x
+ * @param $y
+ *
+ * @return int
+ */
+ protected function getCol($image, $x, $y, $background)
+ {
+ $L = imagesx($image);
+ $H = imagesy($image);
+ if ($x < 0 || $x >= $L || $y < 0 || $y >= $H) {
+ return $background;
+ }
+
+ return imagecolorat($image, $x, $y);
+ }
+
+ /**
+ * @param $col
+ *
+ * @return array
+ */
+ protected function getRGB($col)
+ {
+ return array(
+ (int) ($col >> 16) & 0xff,
+ (int) ($col >> 8) & 0xff,
+ (int) ($col) & 0xff,
+ );
+ }
+
+ /**
+ * Validate the background image path. Return the image type if valid
+ *
+ * @param string $backgroundImage
+ * @return string
+ * @throws Exception
+ */
+ protected function validateBackgroundImage($backgroundImage)
+ {
+ // check if file exists
+ if (!file_exists($backgroundImage)) {
+ $backgroundImageExploded = explode('/', $backgroundImage);
+ $imageFileName = count($backgroundImageExploded) > 1? $backgroundImageExploded[count($backgroundImageExploded)-1] : $backgroundImage;
+
+ throw new Exception('Invalid background image: ' . $imageFileName);
+ }
+
+ // check image type
+ $finfo = finfo_open(FILEINFO_MIME_TYPE); // return mime type ala mimetype extension
+ $imageType = finfo_file($finfo, $backgroundImage);
+ finfo_close($finfo);
+
+ if (!in_array($imageType, $this->allowedBackgroundImageTypes)) {
+ throw new Exception('Invalid background image type! Allowed types are: ' . join(', ', $this->allowedBackgroundImageTypes));
+ }
+
+ return $imageType;
+ }
+
+ /**
+ * Create background image from type
+ *
+ * @param string $backgroundImage
+ * @param string $imageType
+ * @return resource
+ * @throws Exception
+ */
+ protected function createBackgroundImageFromType($backgroundImage, $imageType)
+ {
+ switch ($imageType) {
+ case 'image/jpeg':
+ $image = imagecreatefromjpeg($backgroundImage);
+ break;
+ case 'image/png':
+ $image = imagecreatefrompng($backgroundImage);
+ break;
+ case 'image/gif':
+ $image = imagecreatefromgif($backgroundImage);
+ break;
+
+ default:
+ throw new Exception('Not supported file type for background image!');
+ break;
+ }
+
+ return $image;
+ }
+}
diff --git a/plugins/Captcha/src/Gregwar/Captcha/CaptchaBuilderInterface.php b/plugins/Captcha/src/Gregwar/Captcha/CaptchaBuilderInterface.php
new file mode 100644
index 0000000..bebd070
--- /dev/null
+++ b/plugins/Captcha/src/Gregwar/Captcha/CaptchaBuilderInterface.php
@@ -0,0 +1,29 @@
+
+ * @author Jeremy Livingston
+ */
+class ImageFileHandler
+{
+ /**
+ * Name of folder for captcha images
+ * @var string
+ */
+ protected $imageFolder;
+
+ /**
+ * Absolute path to public web folder
+ * @var string
+ */
+ protected $webPath;
+
+ /**
+ * Frequency of garbage collection in fractions of 1
+ * @var int
+ */
+ protected $gcFreq;
+
+ /**
+ * Maximum age of images in minutes
+ * @var int
+ */
+ protected $expiration;
+
+ /**
+ * @param $imageFolder
+ * @param $webPath
+ * @param $gcFreq
+ * @param $expiration
+ */
+ public function __construct($imageFolder, $webPath, $gcFreq, $expiration)
+ {
+ $this->imageFolder = $imageFolder;
+ $this->webPath = $webPath;
+ $this->gcFreq = $gcFreq;
+ $this->expiration = $expiration;
+ }
+
+ /**
+ * Saves the provided image content as a file
+ *
+ * @param string $contents
+ *
+ * @return string
+ */
+ public function saveAsFile($contents)
+ {
+ $this->createFolderIfMissing();
+
+ $filename = md5(uniqid()) . '.jpg';
+ $filePath = $this->webPath . '/' . $this->imageFolder . '/' . $filename;
+ imagejpeg($contents, $filePath, 15);
+
+ return '/' . $this->imageFolder . '/' . $filename;
+ }
+
+ /**
+ * Randomly runs garbage collection on the image directory
+ *
+ * @return bool
+ */
+ public function collectGarbage()
+ {
+ if (!mt_rand(1, $this->gcFreq) == 1) {
+ return false;
+ }
+
+ $this->createFolderIfMissing();
+
+ $finder = new Finder();
+ $criteria = sprintf('<= now - %s minutes', $this->expiration);
+ $finder->in($this->webPath . '/' . $this->imageFolder)
+ ->date($criteria);
+
+ foreach ($finder->files() as $file) {
+ unlink($file->getPathname());
+ }
+
+ return true;
+ }
+
+ /**
+ * Creates the folder if it doesn't exist
+ */
+ protected function createFolderIfMissing()
+ {
+ if (!file_exists($this->webPath . '/' . $this->imageFolder)) {
+ mkdir($this->webPath . '/' . $this->imageFolder, 0755);
+ }
+ }
+}
diff --git a/plugins/Captcha/src/Gregwar/Captcha/PhraseBuilder.php b/plugins/Captcha/src/Gregwar/Captcha/PhraseBuilder.php
new file mode 100644
index 0000000..aa6ecf1
--- /dev/null
+++ b/plugins/Captcha/src/Gregwar/Captcha/PhraseBuilder.php
@@ -0,0 +1,75 @@
+
+ */
+class PhraseBuilder implements PhraseBuilderInterface
+{
+ /**
+ * @var int
+ */
+ public $length;
+
+ /**
+ * @var string
+ */
+ public $charset;
+ /**
+ * Constructs a PhraseBuilder with given parameters
+ */
+ public function __construct($length = 5, $charset = 'abcdefghijklmnpqrstuvwxyz123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ')
+ {
+ $this->length = $length;
+ $this->charset = $charset;
+ }
+
+ /**
+ * Generates random phrase of given length with given charset
+ */
+ public function build($length = null, $charset = null)
+ {
+ if ($length !== null) {
+ $this->length = $length;
+ }
+ if ($charset !== null) {
+ $this->charset = $charset;
+ }
+
+ $phrase = '';
+ $chars = str_split($this->charset);
+
+ for ($i = 0; $i < $this->length; $i++) {
+ $phrase .= $chars[array_rand($chars)];
+ }
+
+ return $phrase;
+ }
+
+ /**
+ * "Niceize" a code
+ */
+ public function niceize($str)
+ {
+ return self::doNiceize($str);
+ }
+
+ /**
+ * A static helper to niceize
+ */
+ public static function doNiceize($str)
+ {
+ return strtr(strtolower($str), '01', 'ol');
+ }
+
+ /**
+ * A static helper to compare
+ */
+ public static function comparePhrases($str1, $str2)
+ {
+ return self::doNiceize($str1) === self::doNiceize($str2);
+ }
+}
diff --git a/plugins/Captcha/src/Gregwar/Captcha/PhraseBuilderInterface.php b/plugins/Captcha/src/Gregwar/Captcha/PhraseBuilderInterface.php
new file mode 100644
index 0000000..62ec4b0
--- /dev/null
+++ b/plugins/Captcha/src/Gregwar/Captcha/PhraseBuilderInterface.php
@@ -0,0 +1,21 @@
+
+ */
+interface PhraseBuilderInterface
+{
+ /**
+ * Generates random phrase of given length with given charset
+ */
+ public function build();
+
+ /**
+ * "Niceize" a code
+ */
+ public function niceize($str);
+}
diff --git a/plugins/Captcha/vendor/autoload.php b/plugins/Captcha/vendor/autoload.php
new file mode 100644
index 0000000..0d9ec67
--- /dev/null
+++ b/plugins/Captcha/vendor/autoload.php
@@ -0,0 +1,12 @@
+
+ * Jordi Boggiano
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Composer\Autoload;
+
+/**
+ * ClassLoader implements a PSR-0, PSR-4 and classmap class loader.
+ *
+ * $loader = new \Composer\Autoload\ClassLoader();
+ *
+ * // register classes with namespaces
+ * $loader->add('Symfony\Component', __DIR__.'/component');
+ * $loader->add('Symfony', __DIR__.'/framework');
+ *
+ * // activate the autoloader
+ * $loader->register();
+ *
+ * // to enable searching the include path (eg. for PEAR packages)
+ * $loader->setUseIncludePath(true);
+ *
+ * In this example, if you try to use a class in the Symfony\Component
+ * namespace or one of its children (Symfony\Component\Console for instance),
+ * the autoloader will first look for the class under the component/
+ * directory, and it will then fallback to the framework/ directory if not
+ * found before giving up.
+ *
+ * This class is loosely based on the Symfony UniversalClassLoader.
+ *
+ * @author Fabien Potencier
+ * @author Jordi Boggiano
+ * @see https://www.php-fig.org/psr/psr-0/
+ * @see https://www.php-fig.org/psr/psr-4/
+ */
+class ClassLoader
+{
+ /** @var ?string */
+ private $vendorDir;
+
+ // PSR-4
+ /**
+ * @var array[]
+ * @psalm-var array>
+ */
+ private $prefixLengthsPsr4 = array();
+ /**
+ * @var array[]
+ * @psalm-var array>
+ */
+ private $prefixDirsPsr4 = array();
+ /**
+ * @var array[]
+ * @psalm-var array
+ */
+ private $fallbackDirsPsr4 = array();
+
+ // PSR-0
+ /**
+ * @var array[]
+ * @psalm-var array>
+ */
+ private $prefixesPsr0 = array();
+ /**
+ * @var array[]
+ * @psalm-var array
+ */
+ private $fallbackDirsPsr0 = array();
+
+ /** @var bool */
+ private $useIncludePath = false;
+
+ /**
+ * @var string[]
+ * @psalm-var array
+ */
+ private $classMap = array();
+
+ /** @var bool */
+ private $classMapAuthoritative = false;
+
+ /**
+ * @var bool[]
+ * @psalm-var array
+ */
+ private $missingClasses = array();
+
+ /** @var ?string */
+ private $apcuPrefix;
+
+ /**
+ * @var self[]
+ */
+ private static $registeredLoaders = array();
+
+ /**
+ * @param ?string $vendorDir
+ */
+ public function __construct($vendorDir = null)
+ {
+ $this->vendorDir = $vendorDir;
+ }
+
+ /**
+ * @return string[]
+ */
+ public function getPrefixes()
+ {
+ if (!empty($this->prefixesPsr0)) {
+ return call_user_func_array('array_merge', array_values($this->prefixesPsr0));
+ }
+
+ return array();
+ }
+
+ /**
+ * @return array[]
+ * @psalm-return array>
+ */
+ public function getPrefixesPsr4()
+ {
+ return $this->prefixDirsPsr4;
+ }
+
+ /**
+ * @return array[]
+ * @psalm-return array
+ */
+ public function getFallbackDirs()
+ {
+ return $this->fallbackDirsPsr0;
+ }
+
+ /**
+ * @return array[]
+ * @psalm-return array
+ */
+ public function getFallbackDirsPsr4()
+ {
+ return $this->fallbackDirsPsr4;
+ }
+
+ /**
+ * @return string[] Array of classname => path
+ * @psalm-return array
+ */
+ public function getClassMap()
+ {
+ return $this->classMap;
+ }
+
+ /**
+ * @param string[] $classMap Class to filename map
+ * @psalm-param array $classMap
+ *
+ * @return void
+ */
+ public function addClassMap(array $classMap)
+ {
+ if ($this->classMap) {
+ $this->classMap = array_merge($this->classMap, $classMap);
+ } else {
+ $this->classMap = $classMap;
+ }
+ }
+
+ /**
+ * Registers a set of PSR-0 directories for a given prefix, either
+ * appending or prepending to the ones previously set for this prefix.
+ *
+ * @param string $prefix The prefix
+ * @param string[]|string $paths The PSR-0 root directories
+ * @param bool $prepend Whether to prepend the directories
+ *
+ * @return void
+ */
+ public function add($prefix, $paths, $prepend = false)
+ {
+ if (!$prefix) {
+ if ($prepend) {
+ $this->fallbackDirsPsr0 = array_merge(
+ (array) $paths,
+ $this->fallbackDirsPsr0
+ );
+ } else {
+ $this->fallbackDirsPsr0 = array_merge(
+ $this->fallbackDirsPsr0,
+ (array) $paths
+ );
+ }
+
+ return;
+ }
+
+ $first = $prefix[0];
+ if (!isset($this->prefixesPsr0[$first][$prefix])) {
+ $this->prefixesPsr0[$first][$prefix] = (array) $paths;
+
+ return;
+ }
+ if ($prepend) {
+ $this->prefixesPsr0[$first][$prefix] = array_merge(
+ (array) $paths,
+ $this->prefixesPsr0[$first][$prefix]
+ );
+ } else {
+ $this->prefixesPsr0[$first][$prefix] = array_merge(
+ $this->prefixesPsr0[$first][$prefix],
+ (array) $paths
+ );
+ }
+ }
+
+ /**
+ * Registers a set of PSR-4 directories for a given namespace, either
+ * appending or prepending to the ones previously set for this namespace.
+ *
+ * @param string $prefix The prefix/namespace, with trailing '\\'
+ * @param string[]|string $paths The PSR-4 base directories
+ * @param bool $prepend Whether to prepend the directories
+ *
+ * @throws \InvalidArgumentException
+ *
+ * @return void
+ */
+ public function addPsr4($prefix, $paths, $prepend = false)
+ {
+ if (!$prefix) {
+ // Register directories for the root namespace.
+ if ($prepend) {
+ $this->fallbackDirsPsr4 = array_merge(
+ (array) $paths,
+ $this->fallbackDirsPsr4
+ );
+ } else {
+ $this->fallbackDirsPsr4 = array_merge(
+ $this->fallbackDirsPsr4,
+ (array) $paths
+ );
+ }
+ } elseif (!isset($this->prefixDirsPsr4[$prefix])) {
+ // Register directories for a new namespace.
+ $length = strlen($prefix);
+ if ('\\' !== $prefix[$length - 1]) {
+ throw new \InvalidArgumentException("A non-empty PSR-4 prefix must end with a namespace separator.");
+ }
+ $this->prefixLengthsPsr4[$prefix[0]][$prefix] = $length;
+ $this->prefixDirsPsr4[$prefix] = (array) $paths;
+ } elseif ($prepend) {
+ // Prepend directories for an already registered namespace.
+ $this->prefixDirsPsr4[$prefix] = array_merge(
+ (array) $paths,
+ $this->prefixDirsPsr4[$prefix]
+ );
+ } else {
+ // Append directories for an already registered namespace.
+ $this->prefixDirsPsr4[$prefix] = array_merge(
+ $this->prefixDirsPsr4[$prefix],
+ (array) $paths
+ );
+ }
+ }
+
+ /**
+ * Registers a set of PSR-0 directories for a given prefix,
+ * replacing any others previously set for this prefix.
+ *
+ * @param string $prefix The prefix
+ * @param string[]|string $paths The PSR-0 base directories
+ *
+ * @return void
+ */
+ public function set($prefix, $paths)
+ {
+ if (!$prefix) {
+ $this->fallbackDirsPsr0 = (array) $paths;
+ } else {
+ $this->prefixesPsr0[$prefix[0]][$prefix] = (array) $paths;
+ }
+ }
+
+ /**
+ * Registers a set of PSR-4 directories for a given namespace,
+ * replacing any others previously set for this namespace.
+ *
+ * @param string $prefix The prefix/namespace, with trailing '\\'
+ * @param string[]|string $paths The PSR-4 base directories
+ *
+ * @throws \InvalidArgumentException
+ *
+ * @return void
+ */
+ public function setPsr4($prefix, $paths)
+ {
+ if (!$prefix) {
+ $this->fallbackDirsPsr4 = (array) $paths;
+ } else {
+ $length = strlen($prefix);
+ if ('\\' !== $prefix[$length - 1]) {
+ throw new \InvalidArgumentException("A non-empty PSR-4 prefix must end with a namespace separator.");
+ }
+ $this->prefixLengthsPsr4[$prefix[0]][$prefix] = $length;
+ $this->prefixDirsPsr4[$prefix] = (array) $paths;
+ }
+ }
+
+ /**
+ * Turns on searching the include path for class files.
+ *
+ * @param bool $useIncludePath
+ *
+ * @return void
+ */
+ public function setUseIncludePath($useIncludePath)
+ {
+ $this->useIncludePath = $useIncludePath;
+ }
+
+ /**
+ * Can be used to check if the autoloader uses the include path to check
+ * for classes.
+ *
+ * @return bool
+ */
+ public function getUseIncludePath()
+ {
+ return $this->useIncludePath;
+ }
+
+ /**
+ * Turns off searching the prefix and fallback directories for classes
+ * that have not been registered with the class map.
+ *
+ * @param bool $classMapAuthoritative
+ *
+ * @return void
+ */
+ public function setClassMapAuthoritative($classMapAuthoritative)
+ {
+ $this->classMapAuthoritative = $classMapAuthoritative;
+ }
+
+ /**
+ * Should class lookup fail if not found in the current class map?
+ *
+ * @return bool
+ */
+ public function isClassMapAuthoritative()
+ {
+ return $this->classMapAuthoritative;
+ }
+
+ /**
+ * APCu prefix to use to cache found/not-found classes, if the extension is enabled.
+ *
+ * @param string|null $apcuPrefix
+ *
+ * @return void
+ */
+ public function setApcuPrefix($apcuPrefix)
+ {
+ $this->apcuPrefix = function_exists('apcu_fetch') && filter_var(ini_get('apc.enabled'), FILTER_VALIDATE_BOOLEAN) ? $apcuPrefix : null;
+ }
+
+ /**
+ * The APCu prefix in use, or null if APCu caching is not enabled.
+ *
+ * @return string|null
+ */
+ public function getApcuPrefix()
+ {
+ return $this->apcuPrefix;
+ }
+
+ /**
+ * Registers this instance as an autoloader.
+ *
+ * @param bool $prepend Whether to prepend the autoloader or not
+ *
+ * @return void
+ */
+ public function register($prepend = false)
+ {
+ spl_autoload_register(array($this, 'loadClass'), true, $prepend);
+
+ if (null === $this->vendorDir) {
+ return;
+ }
+
+ if ($prepend) {
+ self::$registeredLoaders = array($this->vendorDir => $this) + self::$registeredLoaders;
+ } else {
+ unset(self::$registeredLoaders[$this->vendorDir]);
+ self::$registeredLoaders[$this->vendorDir] = $this;
+ }
+ }
+
+ /**
+ * Unregisters this instance as an autoloader.
+ *
+ * @return void
+ */
+ public function unregister()
+ {
+ spl_autoload_unregister(array($this, 'loadClass'));
+
+ if (null !== $this->vendorDir) {
+ unset(self::$registeredLoaders[$this->vendorDir]);
+ }
+ }
+
+ /**
+ * Loads the given class or interface.
+ *
+ * @param string $class The name of the class
+ * @return true|null True if loaded, null otherwise
+ */
+ public function loadClass($class)
+ {
+ if ($file = $this->findFile($class)) {
+ includeFile($file);
+
+ return true;
+ }
+
+ return null;
+ }
+
+ /**
+ * Finds the path to the file where the class is defined.
+ *
+ * @param string $class The name of the class
+ *
+ * @return string|false The path if found, false otherwise
+ */
+ public function findFile($class)
+ {
+ // class map lookup
+ if (isset($this->classMap[$class])) {
+ return $this->classMap[$class];
+ }
+ if ($this->classMapAuthoritative || isset($this->missingClasses[$class])) {
+ return false;
+ }
+ if (null !== $this->apcuPrefix) {
+ $file = apcu_fetch($this->apcuPrefix.$class, $hit);
+ if ($hit) {
+ return $file;
+ }
+ }
+
+ $file = $this->findFileWithExtension($class, '.php');
+
+ // Search for Hack files if we are running on HHVM
+ if (false === $file && defined('HHVM_VERSION')) {
+ $file = $this->findFileWithExtension($class, '.hh');
+ }
+
+ if (null !== $this->apcuPrefix) {
+ apcu_add($this->apcuPrefix.$class, $file);
+ }
+
+ if (false === $file) {
+ // Remember that this class does not exist.
+ $this->missingClasses[$class] = true;
+ }
+
+ return $file;
+ }
+
+ /**
+ * Returns the currently registered loaders indexed by their corresponding vendor directories.
+ *
+ * @return self[]
+ */
+ public static function getRegisteredLoaders()
+ {
+ return self::$registeredLoaders;
+ }
+
+ /**
+ * @param string $class
+ * @param string $ext
+ * @return string|false
+ */
+ private function findFileWithExtension($class, $ext)
+ {
+ // PSR-4 lookup
+ $logicalPathPsr4 = strtr($class, '\\', DIRECTORY_SEPARATOR) . $ext;
+
+ $first = $class[0];
+ if (isset($this->prefixLengthsPsr4[$first])) {
+ $subPath = $class;
+ while (false !== $lastPos = strrpos($subPath, '\\')) {
+ $subPath = substr($subPath, 0, $lastPos);
+ $search = $subPath . '\\';
+ if (isset($this->prefixDirsPsr4[$search])) {
+ $pathEnd = DIRECTORY_SEPARATOR . substr($logicalPathPsr4, $lastPos + 1);
+ foreach ($this->prefixDirsPsr4[$search] as $dir) {
+ if (file_exists($file = $dir . $pathEnd)) {
+ return $file;
+ }
+ }
+ }
+ }
+ }
+
+ // PSR-4 fallback dirs
+ foreach ($this->fallbackDirsPsr4 as $dir) {
+ if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr4)) {
+ return $file;
+ }
+ }
+
+ // PSR-0 lookup
+ if (false !== $pos = strrpos($class, '\\')) {
+ // namespaced class name
+ $logicalPathPsr0 = substr($logicalPathPsr4, 0, $pos + 1)
+ . strtr(substr($logicalPathPsr4, $pos + 1), '_', DIRECTORY_SEPARATOR);
+ } else {
+ // PEAR-like class name
+ $logicalPathPsr0 = strtr($class, '_', DIRECTORY_SEPARATOR) . $ext;
+ }
+
+ if (isset($this->prefixesPsr0[$first])) {
+ foreach ($this->prefixesPsr0[$first] as $prefix => $dirs) {
+ if (0 === strpos($class, $prefix)) {
+ foreach ($dirs as $dir) {
+ if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr0)) {
+ return $file;
+ }
+ }
+ }
+ }
+ }
+
+ // PSR-0 fallback dirs
+ foreach ($this->fallbackDirsPsr0 as $dir) {
+ if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr0)) {
+ return $file;
+ }
+ }
+
+ // PSR-0 include paths.
+ if ($this->useIncludePath && $file = stream_resolve_include_path($logicalPathPsr0)) {
+ return $file;
+ }
+
+ return false;
+ }
+}
+
+/**
+ * Scope isolated include.
+ *
+ * Prevents access to $this/self from included files.
+ *
+ * @param string $file
+ * @return void
+ * @private
+ */
+function includeFile($file)
+{
+ include $file;
+}
diff --git a/plugins/Captcha/vendor/composer/InstalledVersions.php b/plugins/Captcha/vendor/composer/InstalledVersions.php
new file mode 100644
index 0000000..d85e22a
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/InstalledVersions.php
@@ -0,0 +1,352 @@
+
+ * Jordi Boggiano
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Composer;
+
+use Composer\Autoload\ClassLoader;
+use Composer\Semver\VersionParser;
+
+/**
+ * This class is copied in every Composer installed project and available to all
+ *
+ * See also https://getcomposer.org/doc/07-runtime.md#installed-versions
+ *
+ * To require its presence, you can require `composer-runtime-api ^2.0`
+ *
+ * @final
+ */
+class InstalledVersions
+{
+ /**
+ * @var mixed[]|null
+ * @psalm-var array{root: array{name: string, pretty_version: string, version: string, reference: string|null, type: string, install_path: string, aliases: string[], dev: bool}, versions: array}|array{}|null
+ */
+ private static $installed;
+
+ /**
+ * @var bool|null
+ */
+ private static $canGetVendors;
+
+ /**
+ * @var array[]
+ * @psalm-var array}>
+ */
+ private static $installedByVendor = array();
+
+ /**
+ * Returns a list of all package names which are present, either by being installed, replaced or provided
+ *
+ * @return string[]
+ * @psalm-return list
+ */
+ public static function getInstalledPackages()
+ {
+ $packages = array();
+ foreach (self::getInstalled() as $installed) {
+ $packages[] = array_keys($installed['versions']);
+ }
+
+ if (1 === \count($packages)) {
+ return $packages[0];
+ }
+
+ return array_keys(array_flip(\call_user_func_array('array_merge', $packages)));
+ }
+
+ /**
+ * Returns a list of all package names with a specific type e.g. 'library'
+ *
+ * @param string $type
+ * @return string[]
+ * @psalm-return list
+ */
+ public static function getInstalledPackagesByType($type)
+ {
+ $packagesByType = array();
+
+ foreach (self::getInstalled() as $installed) {
+ foreach ($installed['versions'] as $name => $package) {
+ if (isset($package['type']) && $package['type'] === $type) {
+ $packagesByType[] = $name;
+ }
+ }
+ }
+
+ return $packagesByType;
+ }
+
+ /**
+ * Checks whether the given package is installed
+ *
+ * This also returns true if the package name is provided or replaced by another package
+ *
+ * @param string $packageName
+ * @param bool $includeDevRequirements
+ * @return bool
+ */
+ public static function isInstalled($packageName, $includeDevRequirements = true)
+ {
+ foreach (self::getInstalled() as $installed) {
+ if (isset($installed['versions'][$packageName])) {
+ return $includeDevRequirements || empty($installed['versions'][$packageName]['dev_requirement']);
+ }
+ }
+
+ return false;
+ }
+
+ /**
+ * Checks whether the given package satisfies a version constraint
+ *
+ * e.g. If you want to know whether version 2.3+ of package foo/bar is installed, you would call:
+ *
+ * Composer\InstalledVersions::satisfies(new VersionParser, 'foo/bar', '^2.3')
+ *
+ * @param VersionParser $parser Install composer/semver to have access to this class and functionality
+ * @param string $packageName
+ * @param string|null $constraint A version constraint to check for, if you pass one you have to make sure composer/semver is required by your package
+ * @return bool
+ */
+ public static function satisfies(VersionParser $parser, $packageName, $constraint)
+ {
+ $constraint = $parser->parseConstraints($constraint);
+ $provided = $parser->parseConstraints(self::getVersionRanges($packageName));
+
+ return $provided->matches($constraint);
+ }
+
+ /**
+ * Returns a version constraint representing all the range(s) which are installed for a given package
+ *
+ * It is easier to use this via isInstalled() with the $constraint argument if you need to check
+ * whether a given version of a package is installed, and not just whether it exists
+ *
+ * @param string $packageName
+ * @return string Version constraint usable with composer/semver
+ */
+ public static function getVersionRanges($packageName)
+ {
+ foreach (self::getInstalled() as $installed) {
+ if (!isset($installed['versions'][$packageName])) {
+ continue;
+ }
+
+ $ranges = array();
+ if (isset($installed['versions'][$packageName]['pretty_version'])) {
+ $ranges[] = $installed['versions'][$packageName]['pretty_version'];
+ }
+ if (array_key_exists('aliases', $installed['versions'][$packageName])) {
+ $ranges = array_merge($ranges, $installed['versions'][$packageName]['aliases']);
+ }
+ if (array_key_exists('replaced', $installed['versions'][$packageName])) {
+ $ranges = array_merge($ranges, $installed['versions'][$packageName]['replaced']);
+ }
+ if (array_key_exists('provided', $installed['versions'][$packageName])) {
+ $ranges = array_merge($ranges, $installed['versions'][$packageName]['provided']);
+ }
+
+ return implode(' || ', $ranges);
+ }
+
+ throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
+ }
+
+ /**
+ * @param string $packageName
+ * @return string|null If the package is being replaced or provided but is not really installed, null will be returned as version, use satisfies or getVersionRanges if you need to know if a given version is present
+ */
+ public static function getVersion($packageName)
+ {
+ foreach (self::getInstalled() as $installed) {
+ if (!isset($installed['versions'][$packageName])) {
+ continue;
+ }
+
+ if (!isset($installed['versions'][$packageName]['version'])) {
+ return null;
+ }
+
+ return $installed['versions'][$packageName]['version'];
+ }
+
+ throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
+ }
+
+ /**
+ * @param string $packageName
+ * @return string|null If the package is being replaced or provided but is not really installed, null will be returned as version, use satisfies or getVersionRanges if you need to know if a given version is present
+ */
+ public static function getPrettyVersion($packageName)
+ {
+ foreach (self::getInstalled() as $installed) {
+ if (!isset($installed['versions'][$packageName])) {
+ continue;
+ }
+
+ if (!isset($installed['versions'][$packageName]['pretty_version'])) {
+ return null;
+ }
+
+ return $installed['versions'][$packageName]['pretty_version'];
+ }
+
+ throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
+ }
+
+ /**
+ * @param string $packageName
+ * @return string|null If the package is being replaced or provided but is not really installed, null will be returned as reference
+ */
+ public static function getReference($packageName)
+ {
+ foreach (self::getInstalled() as $installed) {
+ if (!isset($installed['versions'][$packageName])) {
+ continue;
+ }
+
+ if (!isset($installed['versions'][$packageName]['reference'])) {
+ return null;
+ }
+
+ return $installed['versions'][$packageName]['reference'];
+ }
+
+ throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
+ }
+
+ /**
+ * @param string $packageName
+ * @return string|null If the package is being replaced or provided but is not really installed, null will be returned as install path. Packages of type metapackages also have a null install path.
+ */
+ public static function getInstallPath($packageName)
+ {
+ foreach (self::getInstalled() as $installed) {
+ if (!isset($installed['versions'][$packageName])) {
+ continue;
+ }
+
+ return isset($installed['versions'][$packageName]['install_path']) ? $installed['versions'][$packageName]['install_path'] : null;
+ }
+
+ throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
+ }
+
+ /**
+ * @return array
+ * @psalm-return array{name: string, pretty_version: string, version: string, reference: string|null, type: string, install_path: string, aliases: string[], dev: bool}
+ */
+ public static function getRootPackage()
+ {
+ $installed = self::getInstalled();
+
+ return $installed[0]['root'];
+ }
+
+ /**
+ * Returns the raw installed.php data for custom implementations
+ *
+ * @deprecated Use getAllRawData() instead which returns all datasets for all autoloaders present in the process. getRawData only returns the first dataset loaded, which may not be what you expect.
+ * @return array[]
+ * @psalm-return array{root: array{name: string, pretty_version: string, version: string, reference: string|null, type: string, install_path: string, aliases: string[], dev: bool}, versions: array}
+ */
+ public static function getRawData()
+ {
+ @trigger_error('getRawData only returns the first dataset loaded, which may not be what you expect. Use getAllRawData() instead which returns all datasets for all autoloaders present in the process.', E_USER_DEPRECATED);
+
+ if (null === self::$installed) {
+ // only require the installed.php file if this file is loaded from its dumped location,
+ // and not from its source location in the composer/composer package, see https://github.com/composer/composer/issues/9937
+ if (substr(__DIR__, -8, 1) !== 'C' && is_file(__DIR__ . '/installed.php')) {
+ self::$installed = include __DIR__ . '/installed.php';
+ } else {
+ self::$installed = array();
+ }
+ }
+
+ return self::$installed;
+ }
+
+ /**
+ * Returns the raw data of all installed.php which are currently loaded for custom implementations
+ *
+ * @return array[]
+ * @psalm-return list}>
+ */
+ public static function getAllRawData()
+ {
+ return self::getInstalled();
+ }
+
+ /**
+ * Lets you reload the static array from another file
+ *
+ * This is only useful for complex integrations in which a project needs to use
+ * this class but then also needs to execute another project's autoloader in process,
+ * and wants to ensure both projects have access to their version of installed.php.
+ *
+ * A typical case would be PHPUnit, where it would need to make sure it reads all
+ * the data it needs from this class, then call reload() with
+ * `require $CWD/vendor/composer/installed.php` (or similar) as input to make sure
+ * the project in which it runs can then also use this class safely, without
+ * interference between PHPUnit's dependencies and the project's dependencies.
+ *
+ * @param array[] $data A vendor/composer/installed.php data set
+ * @return void
+ *
+ * @psalm-param array{root: array{name: string, pretty_version: string, version: string, reference: string|null, type: string, install_path: string, aliases: string[], dev: bool}, versions: array} $data
+ */
+ public static function reload($data)
+ {
+ self::$installed = $data;
+ self::$installedByVendor = array();
+ }
+
+ /**
+ * @return array[]
+ * @psalm-return list}>
+ */
+ private static function getInstalled()
+ {
+ if (null === self::$canGetVendors) {
+ self::$canGetVendors = method_exists('Composer\Autoload\ClassLoader', 'getRegisteredLoaders');
+ }
+
+ $installed = array();
+
+ if (self::$canGetVendors) {
+ foreach (ClassLoader::getRegisteredLoaders() as $vendorDir => $loader) {
+ if (isset(self::$installedByVendor[$vendorDir])) {
+ $installed[] = self::$installedByVendor[$vendorDir];
+ } elseif (is_file($vendorDir.'/composer/installed.php')) {
+ $installed[] = self::$installedByVendor[$vendorDir] = require $vendorDir.'/composer/installed.php';
+ if (null === self::$installed && strtr($vendorDir.'/composer', '\\', '/') === strtr(__DIR__, '\\', '/')) {
+ self::$installed = $installed[count($installed) - 1];
+ }
+ }
+ }
+ }
+
+ if (null === self::$installed) {
+ // only require the installed.php file if this file is loaded from its dumped location,
+ // and not from its source location in the composer/composer package, see https://github.com/composer/composer/issues/9937
+ if (substr(__DIR__, -8, 1) !== 'C' && is_file(__DIR__ . '/installed.php')) {
+ self::$installed = require __DIR__ . '/installed.php';
+ } else {
+ self::$installed = array();
+ }
+ }
+ $installed[] = self::$installed;
+
+ return $installed;
+ }
+}
diff --git a/plugins/Captcha/vendor/composer/LICENSE b/plugins/Captcha/vendor/composer/LICENSE
new file mode 100644
index 0000000..62ecfd8
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/LICENSE
@@ -0,0 +1,19 @@
+Copyright (c) Nils Adermann, Jordi Boggiano
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is furnished
+to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/plugins/Captcha/vendor/composer/autoload_classmap.php b/plugins/Captcha/vendor/composer/autoload_classmap.php
new file mode 100644
index 0000000..0fb0a2c
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/autoload_classmap.php
@@ -0,0 +1,10 @@
+ $vendorDir . '/composer/InstalledVersions.php',
+);
diff --git a/plugins/Captcha/vendor/composer/autoload_namespaces.php b/plugins/Captcha/vendor/composer/autoload_namespaces.php
new file mode 100644
index 0000000..15a2ff3
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/autoload_namespaces.php
@@ -0,0 +1,9 @@
+ array($vendorDir . '/symfony/finder'),
+ 'Gregwar\\' => array($baseDir . '/src/Gregwar'),
+);
diff --git a/plugins/Captcha/vendor/composer/autoload_real.php b/plugins/Captcha/vendor/composer/autoload_real.php
new file mode 100644
index 0000000..4839060
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/autoload_real.php
@@ -0,0 +1,38 @@
+register(true);
+
+ return $loader;
+ }
+}
diff --git a/plugins/Captcha/vendor/composer/autoload_static.php b/plugins/Captcha/vendor/composer/autoload_static.php
new file mode 100644
index 0000000..6cacb50
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/autoload_static.php
@@ -0,0 +1,44 @@
+
+ array (
+ 'Symfony\\Component\\Finder\\' => 25,
+ ),
+ 'G' =>
+ array (
+ 'Gregwar\\' => 8,
+ ),
+ );
+
+ public static $prefixDirsPsr4 = array (
+ 'Symfony\\Component\\Finder\\' =>
+ array (
+ 0 => __DIR__ . '/..' . '/symfony/finder',
+ ),
+ 'Gregwar\\' =>
+ array (
+ 0 => __DIR__ . '/../..' . '/src/Gregwar',
+ ),
+ );
+
+ public static $classMap = array (
+ 'Composer\\InstalledVersions' => __DIR__ . '/..' . '/composer/InstalledVersions.php',
+ );
+
+ public static function getInitializer(ClassLoader $loader)
+ {
+ return \Closure::bind(function () use ($loader) {
+ $loader->prefixLengthsPsr4 = ComposerStaticInit145df75e95c5bc70c3fe4557e6b6a3f1::$prefixLengthsPsr4;
+ $loader->prefixDirsPsr4 = ComposerStaticInit145df75e95c5bc70c3fe4557e6b6a3f1::$prefixDirsPsr4;
+ $loader->classMap = ComposerStaticInit145df75e95c5bc70c3fe4557e6b6a3f1::$classMap;
+
+ }, null, ClassLoader::class);
+ }
+}
diff --git a/plugins/Captcha/vendor/composer/installed.json b/plugins/Captcha/vendor/composer/installed.json
new file mode 100644
index 0000000..6f990ec
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/installed.json
@@ -0,0 +1,70 @@
+{
+ "packages": [
+ {
+ "name": "symfony/finder",
+ "version": "v6.0.11",
+ "version_normalized": "6.0.11.0",
+ "source": {
+ "type": "git",
+ "url": "https://github.com/symfony/finder.git",
+ "reference": "09cb683ba5720385ea6966e5e06be2a34f2568b1"
+ },
+ "dist": {
+ "type": "zip",
+ "url": "https://api.github.com/repos/symfony/finder/zipball/09cb683ba5720385ea6966e5e06be2a34f2568b1",
+ "reference": "09cb683ba5720385ea6966e5e06be2a34f2568b1",
+ "shasum": ""
+ },
+ "require": {
+ "php": ">=8.0.2"
+ },
+ "time": "2022-07-29T07:39:48+00:00",
+ "type": "library",
+ "installation-source": "dist",
+ "autoload": {
+ "psr-4": {
+ "Symfony\\Component\\Finder\\": ""
+ },
+ "exclude-from-classmap": [
+ "/Tests/"
+ ]
+ },
+ "notification-url": "https://packagist.org/downloads/",
+ "license": [
+ "MIT"
+ ],
+ "authors": [
+ {
+ "name": "Fabien Potencier",
+ "email": "fabien@symfony.com"
+ },
+ {
+ "name": "Symfony Community",
+ "homepage": "https://symfony.com/contributors"
+ }
+ ],
+ "description": "Finds files and directories via an intuitive fluent interface",
+ "homepage": "https://symfony.com",
+ "support": {
+ "source": "https://github.com/symfony/finder/tree/v6.0.11"
+ },
+ "funding": [
+ {
+ "url": "https://symfony.com/sponsor",
+ "type": "custom"
+ },
+ {
+ "url": "https://github.com/fabpot",
+ "type": "github"
+ },
+ {
+ "url": "https://tidelift.com/funding/github/packagist/symfony/symfony",
+ "type": "tidelift"
+ }
+ ],
+ "install-path": "../symfony/finder"
+ }
+ ],
+ "dev": true,
+ "dev-package-names": []
+}
diff --git a/plugins/Captcha/vendor/composer/installed.php b/plugins/Captcha/vendor/composer/installed.php
new file mode 100644
index 0000000..3fb44f3
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/installed.php
@@ -0,0 +1,32 @@
+ array(
+ 'name' => 'gregwar/captcha',
+ 'pretty_version' => '1.0.0+no-version-set',
+ 'version' => '1.0.0.0',
+ 'reference' => NULL,
+ 'type' => 'library',
+ 'install_path' => __DIR__ . '/../../',
+ 'aliases' => array(),
+ 'dev' => true,
+ ),
+ 'versions' => array(
+ 'gregwar/captcha' => array(
+ 'pretty_version' => '1.0.0+no-version-set',
+ 'version' => '1.0.0.0',
+ 'reference' => NULL,
+ 'type' => 'library',
+ 'install_path' => __DIR__ . '/../../',
+ 'aliases' => array(),
+ 'dev_requirement' => false,
+ ),
+ 'symfony/finder' => array(
+ 'pretty_version' => 'v6.0.11',
+ 'version' => '6.0.11.0',
+ 'reference' => '09cb683ba5720385ea6966e5e06be2a34f2568b1',
+ 'type' => 'library',
+ 'install_path' => __DIR__ . '/../symfony/finder',
+ 'aliases' => array(),
+ 'dev_requirement' => false,
+ ),
+ ),
+);
diff --git a/plugins/Captcha/vendor/composer/platform_check.php b/plugins/Captcha/vendor/composer/platform_check.php
new file mode 100644
index 0000000..7621d4f
--- /dev/null
+++ b/plugins/Captcha/vendor/composer/platform_check.php
@@ -0,0 +1,26 @@
+= 50300)) {
+ $issues[] = 'Your Composer dependencies require a PHP version ">= 5.3.0". You are running ' . PHP_VERSION . '.';
+}
+
+if ($issues) {
+ if (!headers_sent()) {
+ header('HTTP/1.1 500 Internal Server Error');
+ }
+ if (!ini_get('display_errors')) {
+ if (PHP_SAPI === 'cli' || PHP_SAPI === 'phpdbg') {
+ fwrite(STDERR, 'Composer detected issues in your platform:' . PHP_EOL.PHP_EOL . implode(PHP_EOL, $issues) . PHP_EOL.PHP_EOL);
+ } elseif (!headers_sent()) {
+ echo 'Composer detected issues in your platform:' . PHP_EOL.PHP_EOL . str_replace('You are running '.PHP_VERSION.'.', '', implode(PHP_EOL, $issues)) . PHP_EOL.PHP_EOL;
+ }
+ }
+ trigger_error(
+ 'Composer detected issues in your platform: ' . implode(' ', $issues),
+ E_USER_ERROR
+ );
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/CHANGELOG.md b/plugins/Captcha/vendor/symfony/finder/CHANGELOG.md
new file mode 100644
index 0000000..9e2fc5a
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/CHANGELOG.md
@@ -0,0 +1,92 @@
+CHANGELOG
+=========
+
+6.0
+---
+
+ * Remove `Comparator::setTarget()` and `Comparator::setOperator()`
+
+5.4.0
+-----
+
+ * Deprecate `Comparator::setTarget()` and `Comparator::setOperator()`
+ * Add a constructor to `Comparator` that allows setting target and operator
+ * Finder's iterator has now `Symfony\Component\Finder\SplFileInfo` inner type specified
+ * Add recursive .gitignore files support
+
+5.0.0
+-----
+
+ * added `$useNaturalSort` argument to `Finder::sortByName()`
+
+4.3.0
+-----
+
+ * added Finder::ignoreVCSIgnored() to ignore files based on rules listed in .gitignore
+
+4.2.0
+-----
+
+ * added $useNaturalSort option to Finder::sortByName() method
+ * the `Finder::sortByName()` method will have a new `$useNaturalSort`
+ argument in version 5.0, not defining it is deprecated
+ * added `Finder::reverseSorting()` to reverse the sorting
+
+4.0.0
+-----
+
+ * removed `ExceptionInterface`
+ * removed `Symfony\Component\Finder\Iterator\FilterIterator`
+
+3.4.0
+-----
+
+ * deprecated `Symfony\Component\Finder\Iterator\FilterIterator`
+ * added Finder::hasResults() method to check if any results were found
+
+3.3.0
+-----
+
+ * added double-star matching to Glob::toRegex()
+
+3.0.0
+-----
+
+ * removed deprecated classes
+
+2.8.0
+-----
+
+ * deprecated adapters and related classes
+
+2.5.0
+-----
+ * added support for GLOB_BRACE in the paths passed to Finder::in()
+
+2.3.0
+-----
+
+ * added a way to ignore unreadable directories (via Finder::ignoreUnreadableDirs())
+ * unified the way subfolders that are not executable are handled by always throwing an AccessDeniedException exception
+
+2.2.0
+-----
+
+ * added Finder::path() and Finder::notPath() methods
+ * added finder adapters to improve performance on specific platforms
+ * added support for wildcard characters (glob patterns) in the paths passed
+ to Finder::in()
+
+2.1.0
+-----
+
+ * added Finder::sortByAccessedTime(), Finder::sortByChangedTime(), and
+ Finder::sortByModifiedTime()
+ * added Countable to Finder
+ * added support for an array of directories as an argument to
+ Finder::exclude()
+ * added searching based on the file content via Finder::contains() and
+ Finder::notContains()
+ * added support for the != operator in the Comparator
+ * [BC BREAK] filter expressions (used for file name and content) are no more
+ considered as regexps but glob patterns when they are enclosed in '*' or '?'
diff --git a/plugins/Captcha/vendor/symfony/finder/Comparator/Comparator.php b/plugins/Captcha/vendor/symfony/finder/Comparator/Comparator.php
new file mode 100644
index 0000000..f1ba97d
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Comparator/Comparator.php
@@ -0,0 +1,68 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Comparator;
+
+/**
+ * @author Fabien Potencier
+ */
+class Comparator
+{
+ private string $target;
+ private string $operator;
+
+ public function __construct(string $target, string $operator = '==')
+ {
+ if (!\in_array($operator, ['>', '<', '>=', '<=', '==', '!='])) {
+ throw new \InvalidArgumentException(sprintf('Invalid operator "%s".', $operator));
+ }
+
+ $this->target = $target;
+ $this->operator = $operator;
+ }
+
+ /**
+ * Gets the target value.
+ */
+ public function getTarget(): string
+ {
+ return $this->target;
+ }
+
+ /**
+ * Gets the comparison operator.
+ */
+ public function getOperator(): string
+ {
+ return $this->operator;
+ }
+
+ /**
+ * Tests against the target.
+ */
+ public function test(mixed $test): bool
+ {
+ switch ($this->operator) {
+ case '>':
+ return $test > $this->target;
+ case '>=':
+ return $test >= $this->target;
+ case '<':
+ return $test < $this->target;
+ case '<=':
+ return $test <= $this->target;
+ case '!=':
+ return $test != $this->target;
+ }
+
+ return $test == $this->target;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Comparator/DateComparator.php b/plugins/Captcha/vendor/symfony/finder/Comparator/DateComparator.php
new file mode 100644
index 0000000..8f651e1
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Comparator/DateComparator.php
@@ -0,0 +1,50 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Comparator;
+
+/**
+ * DateCompare compiles date comparisons.
+ *
+ * @author Fabien Potencier
+ */
+class DateComparator extends Comparator
+{
+ /**
+ * @param string $test A comparison string
+ *
+ * @throws \InvalidArgumentException If the test is not understood
+ */
+ public function __construct(string $test)
+ {
+ if (!preg_match('#^\s*(==|!=|[<>]=?|after|since|before|until)?\s*(.+?)\s*$#i', $test, $matches)) {
+ throw new \InvalidArgumentException(sprintf('Don\'t understand "%s" as a date test.', $test));
+ }
+
+ try {
+ $date = new \DateTime($matches[2]);
+ $target = $date->format('U');
+ } catch (\Exception $e) {
+ throw new \InvalidArgumentException(sprintf('"%s" is not a valid date.', $matches[2]));
+ }
+
+ $operator = $matches[1] ?? '==';
+ if ('since' === $operator || 'after' === $operator) {
+ $operator = '>';
+ }
+
+ if ('until' === $operator || 'before' === $operator) {
+ $operator = '<';
+ }
+
+ parent::__construct($target, $operator);
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Comparator/NumberComparator.php b/plugins/Captcha/vendor/symfony/finder/Comparator/NumberComparator.php
new file mode 100644
index 0000000..ff85d96
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Comparator/NumberComparator.php
@@ -0,0 +1,78 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Comparator;
+
+/**
+ * NumberComparator compiles a simple comparison to an anonymous
+ * subroutine, which you can call with a value to be tested again.
+ *
+ * Now this would be very pointless, if NumberCompare didn't understand
+ * magnitudes.
+ *
+ * The target value may use magnitudes of kilobytes (k, ki),
+ * megabytes (m, mi), or gigabytes (g, gi). Those suffixed
+ * with an i use the appropriate 2**n version in accordance with the
+ * IEC standard: http://physics.nist.gov/cuu/Units/binary.html
+ *
+ * Based on the Perl Number::Compare module.
+ *
+ * @author Fabien Potencier PHP port
+ * @author Richard Clamp Perl version
+ * @copyright 2004-2005 Fabien Potencier
+ * @copyright 2002 Richard Clamp
+ *
+ * @see http://physics.nist.gov/cuu/Units/binary.html
+ */
+class NumberComparator extends Comparator
+{
+ /**
+ * @param string|int $test A comparison string or an integer
+ *
+ * @throws \InvalidArgumentException If the test is not understood
+ */
+ public function __construct(?string $test)
+ {
+ if (null === $test || !preg_match('#^\s*(==|!=|[<>]=?)?\s*([0-9\.]+)\s*([kmg]i?)?\s*$#i', $test, $matches)) {
+ throw new \InvalidArgumentException(sprintf('Don\'t understand "%s" as a number test.', $test ?? 'null'));
+ }
+
+ $target = $matches[2];
+ if (!is_numeric($target)) {
+ throw new \InvalidArgumentException(sprintf('Invalid number "%s".', $target));
+ }
+ if (isset($matches[3])) {
+ // magnitude
+ switch (strtolower($matches[3])) {
+ case 'k':
+ $target *= 1000;
+ break;
+ case 'ki':
+ $target *= 1024;
+ break;
+ case 'm':
+ $target *= 1000000;
+ break;
+ case 'mi':
+ $target *= 1024 * 1024;
+ break;
+ case 'g':
+ $target *= 1000000000;
+ break;
+ case 'gi':
+ $target *= 1024 * 1024 * 1024;
+ break;
+ }
+ }
+
+ parent::__construct($target, $matches[1] ?: '==');
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Exception/AccessDeniedException.php b/plugins/Captcha/vendor/symfony/finder/Exception/AccessDeniedException.php
new file mode 100644
index 0000000..ee195ea
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Exception/AccessDeniedException.php
@@ -0,0 +1,19 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Exception;
+
+/**
+ * @author Jean-François Simon
+ */
+class AccessDeniedException extends \UnexpectedValueException
+{
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Exception/DirectoryNotFoundException.php b/plugins/Captcha/vendor/symfony/finder/Exception/DirectoryNotFoundException.php
new file mode 100644
index 0000000..c6cc0f2
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Exception/DirectoryNotFoundException.php
@@ -0,0 +1,19 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Exception;
+
+/**
+ * @author Andreas Erhard
+ */
+class DirectoryNotFoundException extends \InvalidArgumentException
+{
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Finder.php b/plugins/Captcha/vendor/symfony/finder/Finder.php
new file mode 100644
index 0000000..e5772c4
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Finder.php
@@ -0,0 +1,798 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder;
+
+use Symfony\Component\Finder\Comparator\DateComparator;
+use Symfony\Component\Finder\Comparator\NumberComparator;
+use Symfony\Component\Finder\Exception\DirectoryNotFoundException;
+use Symfony\Component\Finder\Iterator\CustomFilterIterator;
+use Symfony\Component\Finder\Iterator\DateRangeFilterIterator;
+use Symfony\Component\Finder\Iterator\DepthRangeFilterIterator;
+use Symfony\Component\Finder\Iterator\ExcludeDirectoryFilterIterator;
+use Symfony\Component\Finder\Iterator\FilecontentFilterIterator;
+use Symfony\Component\Finder\Iterator\FilenameFilterIterator;
+use Symfony\Component\Finder\Iterator\LazyIterator;
+use Symfony\Component\Finder\Iterator\SizeRangeFilterIterator;
+use Symfony\Component\Finder\Iterator\SortableIterator;
+
+/**
+ * Finder allows to build rules to find files and directories.
+ *
+ * It is a thin wrapper around several specialized iterator classes.
+ *
+ * All rules may be invoked several times.
+ *
+ * All methods return the current Finder object to allow chaining:
+ *
+ * $finder = Finder::create()->files()->name('*.php')->in(__DIR__);
+ *
+ * @author Fabien Potencier
+ *
+ * @implements \IteratorAggregate
+ */
+class Finder implements \IteratorAggregate, \Countable
+{
+ public const IGNORE_VCS_FILES = 1;
+ public const IGNORE_DOT_FILES = 2;
+ public const IGNORE_VCS_IGNORED_FILES = 4;
+
+ private int $mode = 0;
+ private array $names = [];
+ private array $notNames = [];
+ private array $exclude = [];
+ private array $filters = [];
+ private array $depths = [];
+ private array $sizes = [];
+ private bool $followLinks = false;
+ private bool $reverseSorting = false;
+ private \Closure|int|false $sort = false;
+ private int $ignore = 0;
+ private array $dirs = [];
+ private array $dates = [];
+ private array $iterators = [];
+ private array $contains = [];
+ private array $notContains = [];
+ private array $paths = [];
+ private array $notPaths = [];
+ private bool $ignoreUnreadableDirs = false;
+
+ private static array $vcsPatterns = ['.svn', '_svn', 'CVS', '_darcs', '.arch-params', '.monotone', '.bzr', '.git', '.hg'];
+
+ public function __construct()
+ {
+ $this->ignore = static::IGNORE_VCS_FILES | static::IGNORE_DOT_FILES;
+ }
+
+ /**
+ * Creates a new Finder.
+ */
+ public static function create(): static
+ {
+ return new static();
+ }
+
+ /**
+ * Restricts the matching to directories only.
+ *
+ * @return $this
+ */
+ public function directories(): static
+ {
+ $this->mode = Iterator\FileTypeFilterIterator::ONLY_DIRECTORIES;
+
+ return $this;
+ }
+
+ /**
+ * Restricts the matching to files only.
+ *
+ * @return $this
+ */
+ public function files(): static
+ {
+ $this->mode = Iterator\FileTypeFilterIterator::ONLY_FILES;
+
+ return $this;
+ }
+
+ /**
+ * Adds tests for the directory depth.
+ *
+ * Usage:
+ *
+ * $finder->depth('> 1') // the Finder will start matching at level 1.
+ * $finder->depth('< 3') // the Finder will descend at most 3 levels of directories below the starting point.
+ * $finder->depth(['>= 1', '< 3'])
+ *
+ * @param string|int|string[]|int[] $levels The depth level expression or an array of depth levels
+ *
+ * @return $this
+ *
+ * @see DepthRangeFilterIterator
+ * @see NumberComparator
+ */
+ public function depth(string|int|array $levels): static
+ {
+ foreach ((array) $levels as $level) {
+ $this->depths[] = new Comparator\NumberComparator($level);
+ }
+
+ return $this;
+ }
+
+ /**
+ * Adds tests for file dates (last modified).
+ *
+ * The date must be something that strtotime() is able to parse:
+ *
+ * $finder->date('since yesterday');
+ * $finder->date('until 2 days ago');
+ * $finder->date('> now - 2 hours');
+ * $finder->date('>= 2005-10-15');
+ * $finder->date(['>= 2005-10-15', '<= 2006-05-27']);
+ *
+ * @param string|string[] $dates A date range string or an array of date ranges
+ *
+ * @return $this
+ *
+ * @see strtotime
+ * @see DateRangeFilterIterator
+ * @see DateComparator
+ */
+ public function date(string|array $dates): static
+ {
+ foreach ((array) $dates as $date) {
+ $this->dates[] = new Comparator\DateComparator($date);
+ }
+
+ return $this;
+ }
+
+ /**
+ * Adds rules that files must match.
+ *
+ * You can use patterns (delimited with / sign), globs or simple strings.
+ *
+ * $finder->name('*.php')
+ * $finder->name('/\.php$/') // same as above
+ * $finder->name('test.php')
+ * $finder->name(['test.py', 'test.php'])
+ *
+ * @param string|string[] $patterns A pattern (a regexp, a glob, or a string) or an array of patterns
+ *
+ * @return $this
+ *
+ * @see FilenameFilterIterator
+ */
+ public function name(string|array $patterns): static
+ {
+ $this->names = array_merge($this->names, (array) $patterns);
+
+ return $this;
+ }
+
+ /**
+ * Adds rules that files must not match.
+ *
+ * @param string|string[] $patterns A pattern (a regexp, a glob, or a string) or an array of patterns
+ *
+ * @return $this
+ *
+ * @see FilenameFilterIterator
+ */
+ public function notName(string|array $patterns): static
+ {
+ $this->notNames = array_merge($this->notNames, (array) $patterns);
+
+ return $this;
+ }
+
+ /**
+ * Adds tests that file contents must match.
+ *
+ * Strings or PCRE patterns can be used:
+ *
+ * $finder->contains('Lorem ipsum')
+ * $finder->contains('/Lorem ipsum/i')
+ * $finder->contains(['dolor', '/ipsum/i'])
+ *
+ * @param string|string[] $patterns A pattern (string or regexp) or an array of patterns
+ *
+ * @return $this
+ *
+ * @see FilecontentFilterIterator
+ */
+ public function contains(string|array $patterns): static
+ {
+ $this->contains = array_merge($this->contains, (array) $patterns);
+
+ return $this;
+ }
+
+ /**
+ * Adds tests that file contents must not match.
+ *
+ * Strings or PCRE patterns can be used:
+ *
+ * $finder->notContains('Lorem ipsum')
+ * $finder->notContains('/Lorem ipsum/i')
+ * $finder->notContains(['lorem', '/dolor/i'])
+ *
+ * @param string|string[] $patterns A pattern (string or regexp) or an array of patterns
+ *
+ * @return $this
+ *
+ * @see FilecontentFilterIterator
+ */
+ public function notContains(string|array $patterns): static
+ {
+ $this->notContains = array_merge($this->notContains, (array) $patterns);
+
+ return $this;
+ }
+
+ /**
+ * Adds rules that filenames must match.
+ *
+ * You can use patterns (delimited with / sign) or simple strings.
+ *
+ * $finder->path('some/special/dir')
+ * $finder->path('/some\/special\/dir/') // same as above
+ * $finder->path(['some dir', 'another/dir'])
+ *
+ * Use only / as dirname separator.
+ *
+ * @param string|string[] $patterns A pattern (a regexp or a string) or an array of patterns
+ *
+ * @return $this
+ *
+ * @see FilenameFilterIterator
+ */
+ public function path(string|array $patterns): static
+ {
+ $this->paths = array_merge($this->paths, (array) $patterns);
+
+ return $this;
+ }
+
+ /**
+ * Adds rules that filenames must not match.
+ *
+ * You can use patterns (delimited with / sign) or simple strings.
+ *
+ * $finder->notPath('some/special/dir')
+ * $finder->notPath('/some\/special\/dir/') // same as above
+ * $finder->notPath(['some/file.txt', 'another/file.log'])
+ *
+ * Use only / as dirname separator.
+ *
+ * @param string|string[] $patterns A pattern (a regexp or a string) or an array of patterns
+ *
+ * @return $this
+ *
+ * @see FilenameFilterIterator
+ */
+ public function notPath(string|array $patterns): static
+ {
+ $this->notPaths = array_merge($this->notPaths, (array) $patterns);
+
+ return $this;
+ }
+
+ /**
+ * Adds tests for file sizes.
+ *
+ * $finder->size('> 10K');
+ * $finder->size('<= 1Ki');
+ * $finder->size(4);
+ * $finder->size(['> 10K', '< 20K'])
+ *
+ * @param string|int|string[]|int[] $sizes A size range string or an integer or an array of size ranges
+ *
+ * @return $this
+ *
+ * @see SizeRangeFilterIterator
+ * @see NumberComparator
+ */
+ public function size(string|int|array $sizes): static
+ {
+ foreach ((array) $sizes as $size) {
+ $this->sizes[] = new Comparator\NumberComparator($size);
+ }
+
+ return $this;
+ }
+
+ /**
+ * Excludes directories.
+ *
+ * Directories passed as argument must be relative to the ones defined with the `in()` method. For example:
+ *
+ * $finder->in(__DIR__)->exclude('ruby');
+ *
+ * @param string|array $dirs A directory path or an array of directories
+ *
+ * @return $this
+ *
+ * @see ExcludeDirectoryFilterIterator
+ */
+ public function exclude(string|array $dirs): static
+ {
+ $this->exclude = array_merge($this->exclude, (array) $dirs);
+
+ return $this;
+ }
+
+ /**
+ * Excludes "hidden" directories and files (starting with a dot).
+ *
+ * This option is enabled by default.
+ *
+ * @return $this
+ *
+ * @see ExcludeDirectoryFilterIterator
+ */
+ public function ignoreDotFiles(bool $ignoreDotFiles): static
+ {
+ if ($ignoreDotFiles) {
+ $this->ignore |= static::IGNORE_DOT_FILES;
+ } else {
+ $this->ignore &= ~static::IGNORE_DOT_FILES;
+ }
+
+ return $this;
+ }
+
+ /**
+ * Forces the finder to ignore version control directories.
+ *
+ * This option is enabled by default.
+ *
+ * @return $this
+ *
+ * @see ExcludeDirectoryFilterIterator
+ */
+ public function ignoreVCS(bool $ignoreVCS): static
+ {
+ if ($ignoreVCS) {
+ $this->ignore |= static::IGNORE_VCS_FILES;
+ } else {
+ $this->ignore &= ~static::IGNORE_VCS_FILES;
+ }
+
+ return $this;
+ }
+
+ /**
+ * Forces Finder to obey .gitignore and ignore files based on rules listed there.
+ *
+ * This option is disabled by default.
+ *
+ * @return $this
+ */
+ public function ignoreVCSIgnored(bool $ignoreVCSIgnored): static
+ {
+ if ($ignoreVCSIgnored) {
+ $this->ignore |= static::IGNORE_VCS_IGNORED_FILES;
+ } else {
+ $this->ignore &= ~static::IGNORE_VCS_IGNORED_FILES;
+ }
+
+ return $this;
+ }
+
+ /**
+ * Adds VCS patterns.
+ *
+ * @see ignoreVCS()
+ *
+ * @param string|string[] $pattern VCS patterns to ignore
+ */
+ public static function addVCSPattern(string|array $pattern)
+ {
+ foreach ((array) $pattern as $p) {
+ self::$vcsPatterns[] = $p;
+ }
+
+ self::$vcsPatterns = array_unique(self::$vcsPatterns);
+ }
+
+ /**
+ * Sorts files and directories by an anonymous function.
+ *
+ * The anonymous function receives two \SplFileInfo instances to compare.
+ *
+ * This can be slow as all the matching files and directories must be retrieved for comparison.
+ *
+ * @return $this
+ *
+ * @see SortableIterator
+ */
+ public function sort(\Closure $closure): static
+ {
+ $this->sort = $closure;
+
+ return $this;
+ }
+
+ /**
+ * Sorts files and directories by name.
+ *
+ * This can be slow as all the matching files and directories must be retrieved for comparison.
+ *
+ * @return $this
+ *
+ * @see SortableIterator
+ */
+ public function sortByName(bool $useNaturalSort = false): static
+ {
+ $this->sort = $useNaturalSort ? Iterator\SortableIterator::SORT_BY_NAME_NATURAL : Iterator\SortableIterator::SORT_BY_NAME;
+
+ return $this;
+ }
+
+ /**
+ * Sorts files and directories by type (directories before files), then by name.
+ *
+ * This can be slow as all the matching files and directories must be retrieved for comparison.
+ *
+ * @return $this
+ *
+ * @see SortableIterator
+ */
+ public function sortByType(): static
+ {
+ $this->sort = Iterator\SortableIterator::SORT_BY_TYPE;
+
+ return $this;
+ }
+
+ /**
+ * Sorts files and directories by the last accessed time.
+ *
+ * This is the time that the file was last accessed, read or written to.
+ *
+ * This can be slow as all the matching files and directories must be retrieved for comparison.
+ *
+ * @return $this
+ *
+ * @see SortableIterator
+ */
+ public function sortByAccessedTime(): static
+ {
+ $this->sort = Iterator\SortableIterator::SORT_BY_ACCESSED_TIME;
+
+ return $this;
+ }
+
+ /**
+ * Reverses the sorting.
+ *
+ * @return $this
+ */
+ public function reverseSorting(): static
+ {
+ $this->reverseSorting = true;
+
+ return $this;
+ }
+
+ /**
+ * Sorts files and directories by the last inode changed time.
+ *
+ * This is the time that the inode information was last modified (permissions, owner, group or other metadata).
+ *
+ * On Windows, since inode is not available, changed time is actually the file creation time.
+ *
+ * This can be slow as all the matching files and directories must be retrieved for comparison.
+ *
+ * @return $this
+ *
+ * @see SortableIterator
+ */
+ public function sortByChangedTime(): static
+ {
+ $this->sort = Iterator\SortableIterator::SORT_BY_CHANGED_TIME;
+
+ return $this;
+ }
+
+ /**
+ * Sorts files and directories by the last modified time.
+ *
+ * This is the last time the actual contents of the file were last modified.
+ *
+ * This can be slow as all the matching files and directories must be retrieved for comparison.
+ *
+ * @return $this
+ *
+ * @see SortableIterator
+ */
+ public function sortByModifiedTime(): static
+ {
+ $this->sort = Iterator\SortableIterator::SORT_BY_MODIFIED_TIME;
+
+ return $this;
+ }
+
+ /**
+ * Filters the iterator with an anonymous function.
+ *
+ * The anonymous function receives a \SplFileInfo and must return false
+ * to remove files.
+ *
+ * @return $this
+ *
+ * @see CustomFilterIterator
+ */
+ public function filter(\Closure $closure): static
+ {
+ $this->filters[] = $closure;
+
+ return $this;
+ }
+
+ /**
+ * Forces the following of symlinks.
+ *
+ * @return $this
+ */
+ public function followLinks(): static
+ {
+ $this->followLinks = true;
+
+ return $this;
+ }
+
+ /**
+ * Tells finder to ignore unreadable directories.
+ *
+ * By default, scanning unreadable directories content throws an AccessDeniedException.
+ *
+ * @return $this
+ */
+ public function ignoreUnreadableDirs(bool $ignore = true): static
+ {
+ $this->ignoreUnreadableDirs = $ignore;
+
+ return $this;
+ }
+
+ /**
+ * Searches files and directories which match defined rules.
+ *
+ * @param string|string[] $dirs A directory path or an array of directories
+ *
+ * @return $this
+ *
+ * @throws DirectoryNotFoundException if one of the directories does not exist
+ */
+ public function in(string|array $dirs): static
+ {
+ $resolvedDirs = [];
+
+ foreach ((array) $dirs as $dir) {
+ if (is_dir($dir)) {
+ $resolvedDirs[] = [$this->normalizeDir($dir)];
+ } elseif ($glob = glob($dir, (\defined('GLOB_BRACE') ? \GLOB_BRACE : 0) | \GLOB_ONLYDIR | \GLOB_NOSORT)) {
+ sort($glob);
+ $resolvedDirs[] = array_map([$this, 'normalizeDir'], $glob);
+ } else {
+ throw new DirectoryNotFoundException(sprintf('The "%s" directory does not exist.', $dir));
+ }
+ }
+
+ $this->dirs = array_merge($this->dirs, ...$resolvedDirs);
+
+ return $this;
+ }
+
+ /**
+ * Returns an Iterator for the current Finder configuration.
+ *
+ * This method implements the IteratorAggregate interface.
+ *
+ * @return \Iterator
+ *
+ * @throws \LogicException if the in() method has not been called
+ */
+ public function getIterator(): \Iterator
+ {
+ if (0 === \count($this->dirs) && 0 === \count($this->iterators)) {
+ throw new \LogicException('You must call one of in() or append() methods before iterating over a Finder.');
+ }
+
+ if (1 === \count($this->dirs) && 0 === \count($this->iterators)) {
+ $iterator = $this->searchInDirectory($this->dirs[0]);
+
+ if ($this->sort || $this->reverseSorting) {
+ $iterator = (new Iterator\SortableIterator($iterator, $this->sort, $this->reverseSorting))->getIterator();
+ }
+
+ return $iterator;
+ }
+
+ $iterator = new \AppendIterator();
+ foreach ($this->dirs as $dir) {
+ $iterator->append(new \IteratorIterator(new LazyIterator(function () use ($dir) {
+ return $this->searchInDirectory($dir);
+ })));
+ }
+
+ foreach ($this->iterators as $it) {
+ $iterator->append($it);
+ }
+
+ if ($this->sort || $this->reverseSorting) {
+ $iterator = (new Iterator\SortableIterator($iterator, $this->sort, $this->reverseSorting))->getIterator();
+ }
+
+ return $iterator;
+ }
+
+ /**
+ * Appends an existing set of files/directories to the finder.
+ *
+ * The set can be another Finder, an Iterator, an IteratorAggregate, or even a plain array.
+ *
+ * @return $this
+ *
+ * @throws \InvalidArgumentException when the given argument is not iterable
+ */
+ public function append(iterable $iterator): static
+ {
+ if ($iterator instanceof \IteratorAggregate) {
+ $this->iterators[] = $iterator->getIterator();
+ } elseif ($iterator instanceof \Iterator) {
+ $this->iterators[] = $iterator;
+ } elseif (is_iterable($iterator)) {
+ $it = new \ArrayIterator();
+ foreach ($iterator as $file) {
+ $file = $file instanceof \SplFileInfo ? $file : new \SplFileInfo($file);
+ $it[$file->getPathname()] = $file;
+ }
+ $this->iterators[] = $it;
+ } else {
+ throw new \InvalidArgumentException('Finder::append() method wrong argument type.');
+ }
+
+ return $this;
+ }
+
+ /**
+ * Check if any results were found.
+ */
+ public function hasResults(): bool
+ {
+ foreach ($this->getIterator() as $_) {
+ return true;
+ }
+
+ return false;
+ }
+
+ /**
+ * Counts all the results collected by the iterators.
+ */
+ public function count(): int
+ {
+ return iterator_count($this->getIterator());
+ }
+
+ private function searchInDirectory(string $dir): \Iterator
+ {
+ $exclude = $this->exclude;
+ $notPaths = $this->notPaths;
+
+ if (static::IGNORE_VCS_FILES === (static::IGNORE_VCS_FILES & $this->ignore)) {
+ $exclude = array_merge($exclude, self::$vcsPatterns);
+ }
+
+ if (static::IGNORE_DOT_FILES === (static::IGNORE_DOT_FILES & $this->ignore)) {
+ $notPaths[] = '#(^|/)\..+(/|$)#';
+ }
+
+ $minDepth = 0;
+ $maxDepth = \PHP_INT_MAX;
+
+ foreach ($this->depths as $comparator) {
+ switch ($comparator->getOperator()) {
+ case '>':
+ $minDepth = $comparator->getTarget() + 1;
+ break;
+ case '>=':
+ $minDepth = $comparator->getTarget();
+ break;
+ case '<':
+ $maxDepth = $comparator->getTarget() - 1;
+ break;
+ case '<=':
+ $maxDepth = $comparator->getTarget();
+ break;
+ default:
+ $minDepth = $maxDepth = $comparator->getTarget();
+ }
+ }
+
+ $flags = \RecursiveDirectoryIterator::SKIP_DOTS;
+
+ if ($this->followLinks) {
+ $flags |= \RecursiveDirectoryIterator::FOLLOW_SYMLINKS;
+ }
+
+ $iterator = new Iterator\RecursiveDirectoryIterator($dir, $flags, $this->ignoreUnreadableDirs);
+
+ if ($exclude) {
+ $iterator = new Iterator\ExcludeDirectoryFilterIterator($iterator, $exclude);
+ }
+
+ $iterator = new \RecursiveIteratorIterator($iterator, \RecursiveIteratorIterator::SELF_FIRST);
+
+ if ($minDepth > 0 || $maxDepth < \PHP_INT_MAX) {
+ $iterator = new Iterator\DepthRangeFilterIterator($iterator, $minDepth, $maxDepth);
+ }
+
+ if ($this->mode) {
+ $iterator = new Iterator\FileTypeFilterIterator($iterator, $this->mode);
+ }
+
+ if ($this->names || $this->notNames) {
+ $iterator = new Iterator\FilenameFilterIterator($iterator, $this->names, $this->notNames);
+ }
+
+ if ($this->contains || $this->notContains) {
+ $iterator = new Iterator\FilecontentFilterIterator($iterator, $this->contains, $this->notContains);
+ }
+
+ if ($this->sizes) {
+ $iterator = new Iterator\SizeRangeFilterIterator($iterator, $this->sizes);
+ }
+
+ if ($this->dates) {
+ $iterator = new Iterator\DateRangeFilterIterator($iterator, $this->dates);
+ }
+
+ if ($this->filters) {
+ $iterator = new Iterator\CustomFilterIterator($iterator, $this->filters);
+ }
+
+ if ($this->paths || $notPaths) {
+ $iterator = new Iterator\PathFilterIterator($iterator, $this->paths, $notPaths);
+ }
+
+ if (static::IGNORE_VCS_IGNORED_FILES === (static::IGNORE_VCS_IGNORED_FILES & $this->ignore)) {
+ $iterator = new Iterator\VcsIgnoredFilterIterator($iterator, $dir);
+ }
+
+ return $iterator;
+ }
+
+ /**
+ * Normalizes given directory names by removing trailing slashes.
+ *
+ * Excluding: (s)ftp:// or ssh2.(s)ftp:// wrapper
+ */
+ private function normalizeDir(string $dir): string
+ {
+ if ('/' === $dir) {
+ return $dir;
+ }
+
+ $dir = rtrim($dir, '/'.\DIRECTORY_SEPARATOR);
+
+ if (preg_match('#^(ssh2\.)?s?ftp://#', $dir)) {
+ $dir .= '/';
+ }
+
+ return $dir;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Gitignore.php b/plugins/Captcha/vendor/symfony/finder/Gitignore.php
new file mode 100644
index 0000000..d42cca1
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Gitignore.php
@@ -0,0 +1,93 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder;
+
+/**
+ * Gitignore matches against text.
+ *
+ * @author Michael Voříšek
+ * @author Ahmed Abdou
+ */
+class Gitignore
+{
+ /**
+ * Returns a regexp which is the equivalent of the gitignore pattern.
+ *
+ * Format specification: https://git-scm.com/docs/gitignore#_pattern_format
+ */
+ public static function toRegex(string $gitignoreFileContent): string
+ {
+ return self::buildRegex($gitignoreFileContent, false);
+ }
+
+ public static function toRegexMatchingNegatedPatterns(string $gitignoreFileContent): string
+ {
+ return self::buildRegex($gitignoreFileContent, true);
+ }
+
+ private static function buildRegex(string $gitignoreFileContent, bool $inverted): string
+ {
+ $gitignoreFileContent = preg_replace('~(?
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder;
+
+/**
+ * Glob matches globbing patterns against text.
+ *
+ * if match_glob("foo.*", "foo.bar") echo "matched\n";
+ *
+ * // prints foo.bar and foo.baz
+ * $regex = glob_to_regex("foo.*");
+ * for (['foo.bar', 'foo.baz', 'foo', 'bar'] as $t)
+ * {
+ * if (/$regex/) echo "matched: $car\n";
+ * }
+ *
+ * Glob implements glob(3) style matching that can be used to match
+ * against text, rather than fetching names from a filesystem.
+ *
+ * Based on the Perl Text::Glob module.
+ *
+ * @author Fabien Potencier PHP port
+ * @author Richard Clamp Perl version
+ * @copyright 2004-2005 Fabien Potencier
+ * @copyright 2002 Richard Clamp
+ */
+class Glob
+{
+ /**
+ * Returns a regexp which is the equivalent of the glob pattern.
+ */
+ public static function toRegex(string $glob, bool $strictLeadingDot = true, bool $strictWildcardSlash = true, string $delimiter = '#'): string
+ {
+ $firstByte = true;
+ $escaping = false;
+ $inCurlies = 0;
+ $regex = '';
+ $sizeGlob = \strlen($glob);
+ for ($i = 0; $i < $sizeGlob; ++$i) {
+ $car = $glob[$i];
+ if ($firstByte && $strictLeadingDot && '.' !== $car) {
+ $regex .= '(?=[^\.])';
+ }
+
+ $firstByte = '/' === $car;
+
+ if ($firstByte && $strictWildcardSlash && isset($glob[$i + 2]) && '**' === $glob[$i + 1].$glob[$i + 2] && (!isset($glob[$i + 3]) || '/' === $glob[$i + 3])) {
+ $car = '[^/]++/';
+ if (!isset($glob[$i + 3])) {
+ $car .= '?';
+ }
+
+ if ($strictLeadingDot) {
+ $car = '(?=[^\.])'.$car;
+ }
+
+ $car = '/(?:'.$car.')*';
+ $i += 2 + isset($glob[$i + 3]);
+
+ if ('/' === $delimiter) {
+ $car = str_replace('/', '\\/', $car);
+ }
+ }
+
+ if ($delimiter === $car || '.' === $car || '(' === $car || ')' === $car || '|' === $car || '+' === $car || '^' === $car || '$' === $car) {
+ $regex .= "\\$car";
+ } elseif ('*' === $car) {
+ $regex .= $escaping ? '\\*' : ($strictWildcardSlash ? '[^/]*' : '.*');
+ } elseif ('?' === $car) {
+ $regex .= $escaping ? '\\?' : ($strictWildcardSlash ? '[^/]' : '.');
+ } elseif ('{' === $car) {
+ $regex .= $escaping ? '\\{' : '(';
+ if (!$escaping) {
+ ++$inCurlies;
+ }
+ } elseif ('}' === $car && $inCurlies) {
+ $regex .= $escaping ? '}' : ')';
+ if (!$escaping) {
+ --$inCurlies;
+ }
+ } elseif (',' === $car && $inCurlies) {
+ $regex .= $escaping ? ',' : '|';
+ } elseif ('\\' === $car) {
+ if ($escaping) {
+ $regex .= '\\\\';
+ $escaping = false;
+ } else {
+ $escaping = true;
+ }
+
+ continue;
+ } else {
+ $regex .= $car;
+ }
+ $escaping = false;
+ }
+
+ return $delimiter.'^'.$regex.'$'.$delimiter;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/CustomFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/CustomFilterIterator.php
new file mode 100644
index 0000000..82ee81d
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/CustomFilterIterator.php
@@ -0,0 +1,61 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * CustomFilterIterator filters files by applying anonymous functions.
+ *
+ * The anonymous function receives a \SplFileInfo and must return false
+ * to remove files.
+ *
+ * @author Fabien Potencier
+ *
+ * @extends \FilterIterator
+ */
+class CustomFilterIterator extends \FilterIterator
+{
+ private array $filters = [];
+
+ /**
+ * @param \Iterator $iterator The Iterator to filter
+ * @param callable[] $filters An array of PHP callbacks
+ *
+ * @throws \InvalidArgumentException
+ */
+ public function __construct(\Iterator $iterator, array $filters)
+ {
+ foreach ($filters as $filter) {
+ if (!\is_callable($filter)) {
+ throw new \InvalidArgumentException('Invalid PHP callback.');
+ }
+ }
+ $this->filters = $filters;
+
+ parent::__construct($iterator);
+ }
+
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ $fileinfo = $this->current();
+
+ foreach ($this->filters as $filter) {
+ if (false === $filter($fileinfo)) {
+ return false;
+ }
+ }
+
+ return true;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/DateRangeFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/DateRangeFilterIterator.php
new file mode 100644
index 0000000..718d42b
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/DateRangeFilterIterator.php
@@ -0,0 +1,58 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+use Symfony\Component\Finder\Comparator\DateComparator;
+
+/**
+ * DateRangeFilterIterator filters out files that are not in the given date range (last modified dates).
+ *
+ * @author Fabien Potencier
+ *
+ * @extends \FilterIterator
+ */
+class DateRangeFilterIterator extends \FilterIterator
+{
+ private array $comparators = [];
+
+ /**
+ * @param \Iterator $iterator
+ * @param DateComparator[] $comparators
+ */
+ public function __construct(\Iterator $iterator, array $comparators)
+ {
+ $this->comparators = $comparators;
+
+ parent::__construct($iterator);
+ }
+
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ $fileinfo = $this->current();
+
+ if (!file_exists($fileinfo->getPathname())) {
+ return false;
+ }
+
+ $filedate = $fileinfo->getMTime();
+ foreach ($this->comparators as $compare) {
+ if (!$compare->test($filedate)) {
+ return false;
+ }
+ }
+
+ return true;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/DepthRangeFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/DepthRangeFilterIterator.php
new file mode 100644
index 0000000..1cddb5f
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/DepthRangeFilterIterator.php
@@ -0,0 +1,48 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * DepthRangeFilterIterator limits the directory depth.
+ *
+ * @author Fabien Potencier
+ *
+ * @template-covariant TKey
+ * @template-covariant TValue
+ *
+ * @extends \FilterIterator
+ */
+class DepthRangeFilterIterator extends \FilterIterator
+{
+ private int $minDepth = 0;
+
+ /**
+ * @param \RecursiveIteratorIterator<\RecursiveIterator> $iterator The Iterator to filter
+ * @param int $minDepth The min depth
+ * @param int $maxDepth The max depth
+ */
+ public function __construct(\RecursiveIteratorIterator $iterator, int $minDepth = 0, int $maxDepth = \PHP_INT_MAX)
+ {
+ $this->minDepth = $minDepth;
+ $iterator->setMaxDepth(\PHP_INT_MAX === $maxDepth ? -1 : $maxDepth);
+
+ parent::__construct($iterator);
+ }
+
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ return $this->getInnerIterator()->getDepth() >= $this->minDepth;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/ExcludeDirectoryFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/ExcludeDirectoryFilterIterator.php
new file mode 100644
index 0000000..467bc19
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/ExcludeDirectoryFilterIterator.php
@@ -0,0 +1,85 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * ExcludeDirectoryFilterIterator filters out directories.
+ *
+ * @author Fabien Potencier
+ *
+ * @extends \FilterIterator
+ * @implements \RecursiveIterator
+ */
+class ExcludeDirectoryFilterIterator extends \FilterIterator implements \RecursiveIterator
+{
+ private \Iterator $iterator;
+ private bool $isRecursive;
+ private array $excludedDirs = [];
+ private ?string $excludedPattern = null;
+
+ /**
+ * @param \Iterator $iterator The Iterator to filter
+ * @param string[] $directories An array of directories to exclude
+ */
+ public function __construct(\Iterator $iterator, array $directories)
+ {
+ $this->iterator = $iterator;
+ $this->isRecursive = $iterator instanceof \RecursiveIterator;
+ $patterns = [];
+ foreach ($directories as $directory) {
+ $directory = rtrim($directory, '/');
+ if (!$this->isRecursive || str_contains($directory, '/')) {
+ $patterns[] = preg_quote($directory, '#');
+ } else {
+ $this->excludedDirs[$directory] = true;
+ }
+ }
+ if ($patterns) {
+ $this->excludedPattern = '#(?:^|/)(?:'.implode('|', $patterns).')(?:/|$)#';
+ }
+
+ parent::__construct($iterator);
+ }
+
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ if ($this->isRecursive && isset($this->excludedDirs[$this->getFilename()]) && $this->isDir()) {
+ return false;
+ }
+
+ if ($this->excludedPattern) {
+ $path = $this->isDir() ? $this->current()->getRelativePathname() : $this->current()->getRelativePath();
+ $path = str_replace('\\', '/', $path);
+
+ return !preg_match($this->excludedPattern, $path);
+ }
+
+ return true;
+ }
+
+ public function hasChildren(): bool
+ {
+ return $this->isRecursive && $this->iterator->hasChildren();
+ }
+
+ public function getChildren(): self
+ {
+ $children = new self($this->iterator->getChildren(), []);
+ $children->excludedDirs = $this->excludedDirs;
+ $children->excludedPattern = $this->excludedPattern;
+
+ return $children;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/FileTypeFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/FileTypeFilterIterator.php
new file mode 100644
index 0000000..2ed48fb
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/FileTypeFilterIterator.php
@@ -0,0 +1,53 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * FileTypeFilterIterator only keeps files, directories, or both.
+ *
+ * @author Fabien Potencier
+ *
+ * @extends \FilterIterator
+ */
+class FileTypeFilterIterator extends \FilterIterator
+{
+ public const ONLY_FILES = 1;
+ public const ONLY_DIRECTORIES = 2;
+
+ private int $mode;
+
+ /**
+ * @param \Iterator $iterator The Iterator to filter
+ * @param int $mode The mode (self::ONLY_FILES or self::ONLY_DIRECTORIES)
+ */
+ public function __construct(\Iterator $iterator, int $mode)
+ {
+ $this->mode = $mode;
+
+ parent::__construct($iterator);
+ }
+
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ $fileinfo = $this->current();
+ if (self::ONLY_DIRECTORIES === (self::ONLY_DIRECTORIES & $this->mode) && $fileinfo->isFile()) {
+ return false;
+ } elseif (self::ONLY_FILES === (self::ONLY_FILES & $this->mode) && $fileinfo->isDir()) {
+ return false;
+ }
+
+ return true;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/FilecontentFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/FilecontentFilterIterator.php
new file mode 100644
index 0000000..eaa7a5d
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/FilecontentFilterIterator.php
@@ -0,0 +1,56 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * FilecontentFilterIterator filters files by their contents using patterns (regexps or strings).
+ *
+ * @author Fabien Potencier
+ * @author Włodzimierz Gajda
+ *
+ * @extends MultiplePcreFilterIterator
+ */
+class FilecontentFilterIterator extends MultiplePcreFilterIterator
+{
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ if (!$this->matchRegexps && !$this->noMatchRegexps) {
+ return true;
+ }
+
+ $fileinfo = $this->current();
+
+ if ($fileinfo->isDir() || !$fileinfo->isReadable()) {
+ return false;
+ }
+
+ $content = $fileinfo->getContents();
+ if (!$content) {
+ return false;
+ }
+
+ return $this->isAccepted($content);
+ }
+
+ /**
+ * Converts string to regexp if necessary.
+ *
+ * @param string $str Pattern: string or regexp
+ */
+ protected function toRegex(string $str): string
+ {
+ return $this->isRegex($str) ? $str : '/'.preg_quote($str, '/').'/';
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/FilenameFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/FilenameFilterIterator.php
new file mode 100644
index 0000000..05d9535
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/FilenameFilterIterator.php
@@ -0,0 +1,45 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+use Symfony\Component\Finder\Glob;
+
+/**
+ * FilenameFilterIterator filters files by patterns (a regexp, a glob, or a string).
+ *
+ * @author Fabien Potencier
+ *
+ * @extends MultiplePcreFilterIterator
+ */
+class FilenameFilterIterator extends MultiplePcreFilterIterator
+{
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ return $this->isAccepted($this->current()->getFilename());
+ }
+
+ /**
+ * Converts glob to regexp.
+ *
+ * PCRE patterns are left unchanged.
+ * Glob strings are transformed with Glob::toRegex().
+ *
+ * @param string $str Pattern: glob or regexp
+ */
+ protected function toRegex(string $str): string
+ {
+ return $this->isRegex($str) ? $str : Glob::toRegex($str);
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/LazyIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/LazyIterator.php
new file mode 100644
index 0000000..71c4be8
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/LazyIterator.php
@@ -0,0 +1,32 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * @author Jérémy Derussé
+ *
+ * @internal
+ */
+class LazyIterator implements \IteratorAggregate
+{
+ private \Closure $iteratorFactory;
+
+ public function __construct(callable $iteratorFactory)
+ {
+ $this->iteratorFactory = $iteratorFactory instanceof \Closure ? $iteratorFactory : \Closure::fromCallable($iteratorFactory);
+ }
+
+ public function getIterator(): \Traversable
+ {
+ yield from ($this->iteratorFactory)();
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/MultiplePcreFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/MultiplePcreFilterIterator.php
new file mode 100644
index 0000000..1e9e7ff
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/MultiplePcreFilterIterator.php
@@ -0,0 +1,111 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * MultiplePcreFilterIterator filters files using patterns (regexps, globs or strings).
+ *
+ * @author Fabien Potencier
+ *
+ * @template-covariant TKey
+ * @template-covariant TValue
+ *
+ * @extends \FilterIterator
+ */
+abstract class MultiplePcreFilterIterator extends \FilterIterator
+{
+ protected $matchRegexps = [];
+ protected $noMatchRegexps = [];
+
+ /**
+ * @param \Iterator $iterator The Iterator to filter
+ * @param string[] $matchPatterns An array of patterns that need to match
+ * @param string[] $noMatchPatterns An array of patterns that need to not match
+ */
+ public function __construct(\Iterator $iterator, array $matchPatterns, array $noMatchPatterns)
+ {
+ foreach ($matchPatterns as $pattern) {
+ $this->matchRegexps[] = $this->toRegex($pattern);
+ }
+
+ foreach ($noMatchPatterns as $pattern) {
+ $this->noMatchRegexps[] = $this->toRegex($pattern);
+ }
+
+ parent::__construct($iterator);
+ }
+
+ /**
+ * Checks whether the string is accepted by the regex filters.
+ *
+ * If there is no regexps defined in the class, this method will accept the string.
+ * Such case can be handled by child classes before calling the method if they want to
+ * apply a different behavior.
+ */
+ protected function isAccepted(string $string): bool
+ {
+ // should at least not match one rule to exclude
+ foreach ($this->noMatchRegexps as $regex) {
+ if (preg_match($regex, $string)) {
+ return false;
+ }
+ }
+
+ // should at least match one rule
+ if ($this->matchRegexps) {
+ foreach ($this->matchRegexps as $regex) {
+ if (preg_match($regex, $string)) {
+ return true;
+ }
+ }
+
+ return false;
+ }
+
+ // If there is no match rules, the file is accepted
+ return true;
+ }
+
+ /**
+ * Checks whether the string is a regex.
+ */
+ protected function isRegex(string $str): bool
+ {
+ $availableModifiers = 'imsxuADU';
+
+ if (\PHP_VERSION_ID >= 80200) {
+ $availableModifiers .= 'n';
+ }
+
+ if (preg_match('/^(.{3,}?)['.$availableModifiers.']*$/', $str, $m)) {
+ $start = substr($m[1], 0, 1);
+ $end = substr($m[1], -1);
+
+ if ($start === $end) {
+ return !preg_match('/[*?[:alnum:] \\\\]/', $start);
+ }
+
+ foreach ([['{', '}'], ['(', ')'], ['[', ']'], ['<', '>']] as $delimiters) {
+ if ($start === $delimiters[0] && $end === $delimiters[1]) {
+ return true;
+ }
+ }
+ }
+
+ return false;
+ }
+
+ /**
+ * Converts string into regexp.
+ */
+ abstract protected function toRegex(string $str): string;
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/PathFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/PathFilterIterator.php
new file mode 100644
index 0000000..bfe402a
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/PathFilterIterator.php
@@ -0,0 +1,54 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * PathFilterIterator filters files by path patterns (e.g. some/special/dir).
+ *
+ * @author Fabien Potencier
+ * @author Włodzimierz Gajda
+ *
+ * @extends MultiplePcreFilterIterator
+ */
+class PathFilterIterator extends MultiplePcreFilterIterator
+{
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ $filename = $this->current()->getRelativePathname();
+
+ if ('\\' === \DIRECTORY_SEPARATOR) {
+ $filename = str_replace('\\', '/', $filename);
+ }
+
+ return $this->isAccepted($filename);
+ }
+
+ /**
+ * Converts strings to regexp.
+ *
+ * PCRE patterns are left unchanged.
+ *
+ * Default conversion:
+ * 'lorem/ipsum/dolor' ==> 'lorem\/ipsum\/dolor/'
+ *
+ * Use only / as directory separator (on Windows also).
+ *
+ * @param string $str Pattern: regexp or dirname
+ */
+ protected function toRegex(string $str): string
+ {
+ return $this->isRegex($str) ? $str : '/'.preg_quote($str, '/').'/';
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/RecursiveDirectoryIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/RecursiveDirectoryIterator.php
new file mode 100644
index 0000000..4c9779f
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/RecursiveDirectoryIterator.php
@@ -0,0 +1,145 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+use Symfony\Component\Finder\Exception\AccessDeniedException;
+use Symfony\Component\Finder\SplFileInfo;
+
+/**
+ * Extends the \RecursiveDirectoryIterator to support relative paths.
+ *
+ * @author Victor Berchet
+ */
+class RecursiveDirectoryIterator extends \RecursiveDirectoryIterator
+{
+ private bool $ignoreUnreadableDirs;
+ private ?bool $rewindable = null;
+
+ // these 3 properties take part of the performance optimization to avoid redoing the same work in all iterations
+ private string $rootPath;
+ private string $subPath;
+ private string $directorySeparator = '/';
+
+ /**
+ * @throws \RuntimeException
+ */
+ public function __construct(string $path, int $flags, bool $ignoreUnreadableDirs = false)
+ {
+ if ($flags & (self::CURRENT_AS_PATHNAME | self::CURRENT_AS_SELF)) {
+ throw new \RuntimeException('This iterator only support returning current as fileinfo.');
+ }
+
+ parent::__construct($path, $flags);
+ $this->ignoreUnreadableDirs = $ignoreUnreadableDirs;
+ $this->rootPath = $path;
+ if ('/' !== \DIRECTORY_SEPARATOR && !($flags & self::UNIX_PATHS)) {
+ $this->directorySeparator = \DIRECTORY_SEPARATOR;
+ }
+ }
+
+ /**
+ * Return an instance of SplFileInfo with support for relative paths.
+ */
+ public function current(): SplFileInfo
+ {
+ // the logic here avoids redoing the same work in all iterations
+
+ if (!isset($this->subPath)) {
+ $this->subPath = $this->getSubPath();
+ }
+ $subPathname = $this->subPath;
+ if ('' !== $subPathname) {
+ $subPathname .= $this->directorySeparator;
+ }
+ $subPathname .= $this->getFilename();
+
+ if ('/' !== $basePath = $this->rootPath) {
+ $basePath .= $this->directorySeparator;
+ }
+
+ return new SplFileInfo($basePath.$subPathname, $this->subPath, $subPathname);
+ }
+
+ public function hasChildren(bool $allowLinks = false): bool
+ {
+ $hasChildren = parent::hasChildren($allowLinks);
+
+ if (!$hasChildren || !$this->ignoreUnreadableDirs) {
+ return $hasChildren;
+ }
+
+ try {
+ parent::getChildren();
+
+ return true;
+ } catch (\UnexpectedValueException $e) {
+ // If directory is unreadable and finder is set to ignore it, skip children
+ return false;
+ }
+ }
+
+ /**
+ * @throws AccessDeniedException
+ */
+ public function getChildren(): \RecursiveDirectoryIterator
+ {
+ try {
+ $children = parent::getChildren();
+
+ if ($children instanceof self) {
+ // parent method will call the constructor with default arguments, so unreadable dirs won't be ignored anymore
+ $children->ignoreUnreadableDirs = $this->ignoreUnreadableDirs;
+
+ // performance optimization to avoid redoing the same work in all children
+ $children->rewindable = &$this->rewindable;
+ $children->rootPath = $this->rootPath;
+ }
+
+ return $children;
+ } catch (\UnexpectedValueException $e) {
+ throw new AccessDeniedException($e->getMessage(), $e->getCode(), $e);
+ }
+ }
+
+ /**
+ * Do nothing for non rewindable stream.
+ */
+ public function rewind(): void
+ {
+ if (false === $this->isRewindable()) {
+ return;
+ }
+
+ parent::rewind();
+ }
+
+ /**
+ * Checks if the stream is rewindable.
+ */
+ public function isRewindable(): bool
+ {
+ if (null !== $this->rewindable) {
+ return $this->rewindable;
+ }
+
+ if (false !== $stream = @opendir($this->getPath())) {
+ $infos = stream_get_meta_data($stream);
+ closedir($stream);
+
+ if ($infos['seekable']) {
+ return $this->rewindable = true;
+ }
+ }
+
+ return $this->rewindable = false;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/SizeRangeFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/SizeRangeFilterIterator.php
new file mode 100644
index 0000000..925830a
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/SizeRangeFilterIterator.php
@@ -0,0 +1,57 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+use Symfony\Component\Finder\Comparator\NumberComparator;
+
+/**
+ * SizeRangeFilterIterator filters out files that are not in the given size range.
+ *
+ * @author Fabien Potencier
+ *
+ * @extends \FilterIterator
+ */
+class SizeRangeFilterIterator extends \FilterIterator
+{
+ private array $comparators = [];
+
+ /**
+ * @param \Iterator $iterator
+ * @param NumberComparator[] $comparators
+ */
+ public function __construct(\Iterator $iterator, array $comparators)
+ {
+ $this->comparators = $comparators;
+
+ parent::__construct($iterator);
+ }
+
+ /**
+ * Filters the iterator values.
+ */
+ public function accept(): bool
+ {
+ $fileinfo = $this->current();
+ if (!$fileinfo->isFile()) {
+ return true;
+ }
+
+ $filesize = $fileinfo->getSize();
+ foreach ($this->comparators as $compare) {
+ if (!$compare->test($filesize)) {
+ return false;
+ }
+ }
+
+ return true;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/SortableIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/SortableIterator.php
new file mode 100644
index 0000000..b6c34b6
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/SortableIterator.php
@@ -0,0 +1,100 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+/**
+ * SortableIterator applies a sort on a given Iterator.
+ *
+ * @author Fabien Potencier
+ *
+ * @implements \IteratorAggregate
+ */
+class SortableIterator implements \IteratorAggregate
+{
+ public const SORT_BY_NONE = 0;
+ public const SORT_BY_NAME = 1;
+ public const SORT_BY_TYPE = 2;
+ public const SORT_BY_ACCESSED_TIME = 3;
+ public const SORT_BY_CHANGED_TIME = 4;
+ public const SORT_BY_MODIFIED_TIME = 5;
+ public const SORT_BY_NAME_NATURAL = 6;
+
+ private \Traversable $iterator;
+ private \Closure|int $sort;
+
+ /**
+ * @param \Traversable $iterator
+ * @param int|callable $sort The sort type (SORT_BY_NAME, SORT_BY_TYPE, or a PHP callback)
+ *
+ * @throws \InvalidArgumentException
+ */
+ public function __construct(\Traversable $iterator, int|callable $sort, bool $reverseOrder = false)
+ {
+ $this->iterator = $iterator;
+ $order = $reverseOrder ? -1 : 1;
+
+ if (self::SORT_BY_NAME === $sort) {
+ $this->sort = static function (\SplFileInfo $a, \SplFileInfo $b) use ($order) {
+ return $order * strcmp($a->getRealPath() ?: $a->getPathname(), $b->getRealPath() ?: $b->getPathname());
+ };
+ } elseif (self::SORT_BY_NAME_NATURAL === $sort) {
+ $this->sort = static function (\SplFileInfo $a, \SplFileInfo $b) use ($order) {
+ return $order * strnatcmp($a->getRealPath() ?: $a->getPathname(), $b->getRealPath() ?: $b->getPathname());
+ };
+ } elseif (self::SORT_BY_TYPE === $sort) {
+ $this->sort = static function (\SplFileInfo $a, \SplFileInfo $b) use ($order) {
+ if ($a->isDir() && $b->isFile()) {
+ return -$order;
+ } elseif ($a->isFile() && $b->isDir()) {
+ return $order;
+ }
+
+ return $order * strcmp($a->getRealPath() ?: $a->getPathname(), $b->getRealPath() ?: $b->getPathname());
+ };
+ } elseif (self::SORT_BY_ACCESSED_TIME === $sort) {
+ $this->sort = static function (\SplFileInfo $a, \SplFileInfo $b) use ($order) {
+ return $order * ($a->getATime() - $b->getATime());
+ };
+ } elseif (self::SORT_BY_CHANGED_TIME === $sort) {
+ $this->sort = static function (\SplFileInfo $a, \SplFileInfo $b) use ($order) {
+ return $order * ($a->getCTime() - $b->getCTime());
+ };
+ } elseif (self::SORT_BY_MODIFIED_TIME === $sort) {
+ $this->sort = static function (\SplFileInfo $a, \SplFileInfo $b) use ($order) {
+ return $order * ($a->getMTime() - $b->getMTime());
+ };
+ } elseif (self::SORT_BY_NONE === $sort) {
+ $this->sort = $order;
+ } elseif (\is_callable($sort)) {
+ $this->sort = $reverseOrder ? static function (\SplFileInfo $a, \SplFileInfo $b) use ($sort) { return -$sort($a, $b); } : \Closure::fromCallable($sort);
+ } else {
+ throw new \InvalidArgumentException('The SortableIterator takes a PHP callable or a valid built-in sort algorithm as an argument.');
+ }
+ }
+
+ public function getIterator(): \Traversable
+ {
+ if (1 === $this->sort) {
+ return $this->iterator;
+ }
+
+ $array = iterator_to_array($this->iterator, true);
+
+ if (-1 === $this->sort) {
+ $array = array_reverse($array);
+ } else {
+ uasort($array, $this->sort);
+ }
+
+ return new \ArrayIterator($array);
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/Iterator/VcsIgnoredFilterIterator.php b/plugins/Captcha/vendor/symfony/finder/Iterator/VcsIgnoredFilterIterator.php
new file mode 100644
index 0000000..e27158c
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/Iterator/VcsIgnoredFilterIterator.php
@@ -0,0 +1,151 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder\Iterator;
+
+use Symfony\Component\Finder\Gitignore;
+
+final class VcsIgnoredFilterIterator extends \FilterIterator
+{
+ /**
+ * @var string
+ */
+ private $baseDir;
+
+ /**
+ * @var array
+ */
+ private $gitignoreFilesCache = [];
+
+ /**
+ * @var array
+ */
+ private $ignoredPathsCache = [];
+
+ public function __construct(\Iterator $iterator, string $baseDir)
+ {
+ $this->baseDir = $this->normalizePath($baseDir);
+
+ parent::__construct($iterator);
+ }
+
+ public function accept(): bool
+ {
+ $file = $this->current();
+
+ $fileRealPath = $this->normalizePath($file->getRealPath());
+
+ return !$this->isIgnored($fileRealPath);
+ }
+
+ private function isIgnored(string $fileRealPath): bool
+ {
+ if (is_dir($fileRealPath) && !str_ends_with($fileRealPath, '/')) {
+ $fileRealPath .= '/';
+ }
+
+ if (isset($this->ignoredPathsCache[$fileRealPath])) {
+ return $this->ignoredPathsCache[$fileRealPath];
+ }
+
+ $ignored = false;
+
+ foreach ($this->parentsDirectoryDownward($fileRealPath) as $parentDirectory) {
+ if ($this->isIgnored($parentDirectory)) {
+ // rules in ignored directories are ignored, no need to check further.
+ break;
+ }
+
+ $fileRelativePath = substr($fileRealPath, \strlen($parentDirectory) + 1);
+
+ if (null === $regexps = $this->readGitignoreFile("{$parentDirectory}/.gitignore")) {
+ continue;
+ }
+
+ [$exclusionRegex, $inclusionRegex] = $regexps;
+
+ if (preg_match($exclusionRegex, $fileRelativePath)) {
+ $ignored = true;
+
+ continue;
+ }
+
+ if (preg_match($inclusionRegex, $fileRelativePath)) {
+ $ignored = false;
+ }
+ }
+
+ return $this->ignoredPathsCache[$fileRealPath] = $ignored;
+ }
+
+ /**
+ * @return list
+ */
+ private function parentsDirectoryDownward(string $fileRealPath): array
+ {
+ $parentDirectories = [];
+
+ $parentDirectory = $fileRealPath;
+
+ while (true) {
+ $newParentDirectory = \dirname($parentDirectory);
+
+ // dirname('/') = '/'
+ if ($newParentDirectory === $parentDirectory) {
+ break;
+ }
+
+ $parentDirectory = $newParentDirectory;
+
+ if (0 !== strpos($parentDirectory, $this->baseDir)) {
+ break;
+ }
+
+ $parentDirectories[] = $parentDirectory;
+ }
+
+ return array_reverse($parentDirectories);
+ }
+
+ /**
+ * @return array{0: string, 1: string}|null
+ */
+ private function readGitignoreFile(string $path): ?array
+ {
+ if (\array_key_exists($path, $this->gitignoreFilesCache)) {
+ return $this->gitignoreFilesCache[$path];
+ }
+
+ if (!file_exists($path)) {
+ return $this->gitignoreFilesCache[$path] = null;
+ }
+
+ if (!is_file($path) || !is_readable($path)) {
+ throw new \RuntimeException("The \"ignoreVCSIgnored\" option cannot be used by the Finder as the \"{$path}\" file is not readable.");
+ }
+
+ $gitignoreFileContent = file_get_contents($path);
+
+ return $this->gitignoreFilesCache[$path] = [
+ Gitignore::toRegex($gitignoreFileContent),
+ Gitignore::toRegexMatchingNegatedPatterns($gitignoreFileContent),
+ ];
+ }
+
+ private function normalizePath(string $path): string
+ {
+ if ('\\' === \DIRECTORY_SEPARATOR) {
+ return str_replace('\\', '/', $path);
+ }
+
+ return $path;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/LICENSE b/plugins/Captcha/vendor/symfony/finder/LICENSE
new file mode 100644
index 0000000..88bf75b
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/LICENSE
@@ -0,0 +1,19 @@
+Copyright (c) 2004-2022 Fabien Potencier
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is furnished
+to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/plugins/Captcha/vendor/symfony/finder/README.md b/plugins/Captcha/vendor/symfony/finder/README.md
new file mode 100644
index 0000000..22bdeb9
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/README.md
@@ -0,0 +1,14 @@
+Finder Component
+================
+
+The Finder component finds files and directories via an intuitive fluent
+interface.
+
+Resources
+---------
+
+ * [Documentation](https://symfony.com/doc/current/components/finder.html)
+ * [Contributing](https://symfony.com/doc/current/contributing/index.html)
+ * [Report issues](https://github.com/symfony/symfony/issues) and
+ [send Pull Requests](https://github.com/symfony/symfony/pulls)
+ in the [main Symfony repository](https://github.com/symfony/symfony)
diff --git a/plugins/Captcha/vendor/symfony/finder/SplFileInfo.php b/plugins/Captcha/vendor/symfony/finder/SplFileInfo.php
new file mode 100644
index 0000000..867e8e8
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/SplFileInfo.php
@@ -0,0 +1,82 @@
+
+ *
+ * For the full copyright and license information, please view the LICENSE
+ * file that was distributed with this source code.
+ */
+
+namespace Symfony\Component\Finder;
+
+/**
+ * Extends \SplFileInfo to support relative paths.
+ *
+ * @author Fabien Potencier
+ */
+class SplFileInfo extends \SplFileInfo
+{
+ private string $relativePath;
+ private string $relativePathname;
+
+ /**
+ * @param string $file The file name
+ * @param string $relativePath The relative path
+ * @param string $relativePathname The relative path name
+ */
+ public function __construct(string $file, string $relativePath, string $relativePathname)
+ {
+ parent::__construct($file);
+ $this->relativePath = $relativePath;
+ $this->relativePathname = $relativePathname;
+ }
+
+ /**
+ * Returns the relative path.
+ *
+ * This path does not contain the file name.
+ */
+ public function getRelativePath(): string
+ {
+ return $this->relativePath;
+ }
+
+ /**
+ * Returns the relative path name.
+ *
+ * This path contains the file name.
+ */
+ public function getRelativePathname(): string
+ {
+ return $this->relativePathname;
+ }
+
+ public function getFilenameWithoutExtension(): string
+ {
+ $filename = $this->getFilename();
+
+ return pathinfo($filename, \PATHINFO_FILENAME);
+ }
+
+ /**
+ * Returns the contents of the file.
+ *
+ * @throws \RuntimeException
+ */
+ public function getContents(): string
+ {
+ set_error_handler(function ($type, $msg) use (&$error) { $error = $msg; });
+ try {
+ $content = file_get_contents($this->getPathname());
+ } finally {
+ restore_error_handler();
+ }
+ if (false === $content) {
+ throw new \RuntimeException($error);
+ }
+
+ return $content;
+ }
+}
diff --git a/plugins/Captcha/vendor/symfony/finder/composer.json b/plugins/Captcha/vendor/symfony/finder/composer.json
new file mode 100644
index 0000000..2e4b324
--- /dev/null
+++ b/plugins/Captcha/vendor/symfony/finder/composer.json
@@ -0,0 +1,28 @@
+{
+ "name": "symfony/finder",
+ "type": "library",
+ "description": "Finds files and directories via an intuitive fluent interface",
+ "keywords": [],
+ "homepage": "https://symfony.com",
+ "license": "MIT",
+ "authors": [
+ {
+ "name": "Fabien Potencier",
+ "email": "fabien@symfony.com"
+ },
+ {
+ "name": "Symfony Community",
+ "homepage": "https://symfony.com/contributors"
+ }
+ ],
+ "require": {
+ "php": ">=8.0.2"
+ },
+ "autoload": {
+ "psr-4": { "Symfony\\Component\\Finder\\": "" },
+ "exclude-from-classmap": [
+ "/Tests/"
+ ]
+ },
+ "minimum-stability": "dev"
+}